代码改错#include <iostream> #include <string.h> #include <stdio.h> using namespace std; class String { public: String() {} String(char str[20]); char Str[20]; friend istream& operator>>(istream& in, String& s); friend ostream& operator<<(ostream& out, String& s); }; String::String(char str[20]) { size_t len = strlen(str); strcpy_s(Str,len,str); } istream& operator>>(istream& in, String& s) { char p[20]; in.getline(p, 20); size_t len = strlen(p); strcpy_s(s.Str,len, p); return in; } ostream& operator<<(ostream& out, String& s) { out << s.Str; return out; } template<class TNo, class TScore, int num>//TNo和TScore为参数化类型 class Student { private: TNo StudentID; //参数化类型,存储姓名 TScore score[num]; //参数化类型数组,存储num门课程的分数 public: void Input();//数据的录入 TScore MaxScore(); //查找score的最大值并返回该值 void Update(TScore sscore, int i);//更新学生的第i门课程成绩为sscore void SelectSort(); //采用选择排序法对学生成绩进行升序排列 void Print(); //输出所有学生的信息 }; template<class TNo, class TScore, int num> void Student<TNo,TScore,num>::Input() { cin >> StudentID; for (int i = 0; i < num; i++) { cin >> score[i]; } } template<class TNo, class TScore, int num> TScore Student<TNo, TScore, num>::MaxScore() { TScore x = 0; if (num != 0) { for (int i = 0; i < num; i++) { if (score[i] > x) { x = score[i]; } } } return x; } template<class TNo, class TScore, int num> void Student<TNo, TScore, num>::Update(TScore sscore, int i) { if (i >= 0 && i < num) { score[i] = sscore; } } template<class TNo, class TScore, int num> void Student<TNo, TScore, num>::SelectSort() { for (int i = 0; i < num; i++) { TScore a = score[i]; for (int j = i; j < num; j++) { if (score[j] < a) { a = score[j]; } } score[i] = a; } } template<class TNo, class TScore, int num> void Student<TNo, TScore, num>::Print() { cout << MaxScore() << endl; cout << StudentID << "\t"; for (int i = 0; i < num; i++) { cout << score[i] << "\t"; } } int main(void) { Student <String, float, 3> s; s.Input(); s.SelectSort(); s.Print(); return 0; }
时间: 2024-01-19 08:04:36 浏览: 25
在代码中,第一行和第二行之间应该加一个空格,即将:
```c++
#include <iostream> #include <string.h> #include <stdio.h>
```
改为:
```c++
#include <iostream>
#include <string.h>
#include <stdio.h>
```
另外,函数`String::String(char str[20])`中,`strcpy_s`函数的第一个参数应该是`s.Str`,即将:
```c++
strcpy_s(Str,len,str);
```
改为:
```c++
strcpy_s(s.Str,len,str);
```
最后,在`Student<TNo, TScore, num>::SelectSort()`函数中,应该将`a`初始化为`score[i]`,即将:
```c++
TScore a = score[i];
```
改为:
```c++
TScore a = score[j];
```
改完后的代码如下:
相关问题
#include <stdlib.h> #include <stdio.h> #include <iostream> #include <string>
#include <stdlib.h> 是C语言标准库中的一个头文件,它提供了一些常用的函数和宏定义,用于处理内存分配、随机数生成、字符串转换等操作。
#include <stdio.h> 是C语言标准库中的另一个头文件,它提供了输入输出相关的函数和宏定义,用于读取和写入数据。
#include <iostream> 是C++标准库中的一个头文件,它包含了C++中输入输出流的定义,提供了cin、cout等对象和相关操作符,用于方便地进行输入输出操作。
#include <string> 是C++标准库中的另一个头文件,它定义了字符串类string及其相关操作,提供了方便的字符串处理功能。
这些头文件是编程中常用的头文件,通过包含它们可以使用其中定义的函数、类和宏等功能来完成相应的操作。
#include<iostream>与#include<stdio.h>的区别
#include<iostream> 和#include<stdio.h>是两个不同的头文件引用语句,它们有以下区别:
1. 功能:#include<iostream> 是C++标准库中的头文件,用于输入输出操作,包含了cin、cout等对象和函数的定义。而#include<stdio.h>是C语言标准库中的头文件,用于输入输出操作,包含了printf、scanf等函数的定义。
2. 命名空间:#include<iostream> 引入的是std命名空间,需要使用std::cout、std::cin等来访问其中的元素。而#include<stdio.h>没有命名空间的概念,函数名直接使用即可。
3. 扩展名:#include<iostream> 的头文件没有扩展名,因为它是C++标准库中的头文件。而#include<stdio.h>的头文件扩展名为.h,因为它是C语言标准库中的头文件。
4. 文件位置:#include<iostream> 是C++标准库中的头文件,通常位于编译器的include路径中。而#include<stdio.h>是C语言标准库中的头文件,也位于编译器的include路径中。
总之,#include<iostream> 用于C++程序中,提供了更方便的输入输出操作;而#include<stdio.h> 用于C程序中,提供了基本的输入输出功能。
相关推荐
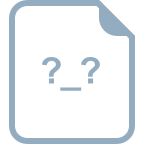














