用C++建立一个windows对话框图形环境应用程序,添加相关编辑框和按钮,点击按钮直接运行固定txt文件(或打开文件对话框,选择 txt文件),统计文件中词的频数,并在图形界面编辑框中显示频数最大10个词,和它的频数。
时间: 2023-07-15 11:15:31 浏览: 125
以下是一个简单的示例代码,实现了你所描述的功能。你可以根据自己的需要进行修改和完善。
```cpp
#include <windows.h>
#include <string>
#include <map>
#include <fstream>
// 定义窗口控件ID
#define IDC_FILEPATH_EDIT 101
#define IDC_OPENFILE_BTN 102
#define IDC_COUNT_BTN 103
#define IDC_RESULT_EDIT 104
// 窗口句柄
HWND hwnd;
// 控件句柄
HWND filePathEdit;
HWND openFileBtn;
HWND countBtn;
HWND resultEdit;
// 打开文件对话框
std::string openFileDialog() {
OPENFILENAME ofn;
char szFile[260] = { 0 };
ZeroMemory(&ofn, sizeof(ofn));
ofn.lStructSize = sizeof(ofn);
ofn.hwndOwner = hwnd;
ofn.lpstrFile = szFile;
ofn.nMaxFile = sizeof(szFile);
ofn.lpstrFilter = "Text Files (*.txt)\0*.txt\0All Files (*.*)\0*.*\0";
ofn.nFilterIndex = 1;
ofn.lpstrInitialDir = NULL;
ofn.Flags = OFN_PATHMUSTEXIST | OFN_FILEMUSTEXIST | OFN_NOCHANGEDIR;
if (GetOpenFileName(&ofn) == TRUE) {
return std::string(szFile);
}
return "";
}
// 统计文本中单词频数
std::map<std::string, int> countWords(std::string filename) {
std::map<std::string, int> wordCount;
std::ifstream file(filename);
if (file.is_open()) {
std::string word;
while (file >> word) {
// 去除单词中的标点符号和空格
std::string cleanWord = "";
for (char c : word) {
if ((c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z')) {
cleanWord += tolower(c);
}
}
if (cleanWord != "") {
if (wordCount.count(cleanWord) == 0) {
wordCount[cleanWord] = 1;
}
else {
wordCount[cleanWord]++;
}
}
}
file.close();
}
return wordCount;
}
// 根据单词频数排序
struct WordFreq {
std::string word;
int freq;
bool operator<(const WordFreq& other) const {
return freq > other.freq;
}
};
// 获取频数前N的单词
std::vector<WordFreq> getTopNWords(std::map<std::string, int> wordCount, int n) {
std::vector<WordFreq> topWords;
for (auto it = wordCount.begin(); it != wordCount.end(); ++it) {
topWords.push_back({ it->first, it->second });
}
std::sort(topWords.begin(), topWords.end());
if (topWords.size() > n) {
topWords.erase(topWords.begin() + n, topWords.end());
}
return topWords;
}
// 更新结果编辑框
void updateResultEdit(std::vector<WordFreq> topWords) {
std::string text = "";
for (WordFreq word : topWords) {
text += word.word + " : " + std::to_string(word.freq) + "\r\n";
}
SetWindowText(resultEdit, text.c_str());
}
// 窗口过程
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam) {
switch (msg) {
case WM_CREATE:
// 创建编辑框
filePathEdit = CreateWindowEx(WS_EX_CLIENTEDGE, "EDIT", "",
WS_CHILD | WS_VISIBLE | ES_LEFT | ES_AUTOHSCROLL,
10, 10, 300, 20, hwnd, (HMENU)IDC_FILEPATH_EDIT, GetModuleHandle(NULL), NULL);
// 创建打开文件按钮
openFileBtn = CreateWindow("BUTTON", "打开文件",
WS_CHILD | WS_VISIBLE | BS_PUSHBUTTON,
320, 10, 80, 20, hwnd, (HMENU)IDC_OPENFILE_BTN, GetModuleHandle(NULL), NULL);
// 创建统计按钮
countBtn = CreateWindow("BUTTON", "统计",
WS_CHILD | WS_VISIBLE | BS_PUSHBUTTON,
410, 10, 80, 20, hwnd, (HMENU)IDC_COUNT_BTN, GetModuleHandle(NULL), NULL);
// 创建结果编辑框
resultEdit = CreateWindowEx(WS_EX_CLIENTEDGE, "EDIT", "",
WS_CHILD | WS_VISIBLE | ES_LEFT | ES_AUTOVSCROLL | ES_MULTILINE | ES_READONLY,
10, 40, 480, 200, hwnd, (HMENU)IDC_RESULT_EDIT, GetModuleHandle(NULL), NULL);
break;
case WM_COMMAND:
switch (LOWORD(wParam)) {
case IDC_OPENFILE_BTN:
// 打开文件对话框
std::string filename = openFileDialog();
if (filename != "") {
SetWindowText(filePathEdit, filename.c_str());
}
break;
case IDC_COUNT_BTN:
// 统计单词频数
std::string filepath = "";
char buffer[256];
GetWindowText(filePathEdit, buffer, sizeof(buffer));
filepath = std::string(buffer);
std::map<std::string, int> wordCount = countWords(filepath);
std::vector<WordFreq> topWords = getTopNWords(wordCount, 10);
updateResultEdit(topWords);
break;
}
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
// 程序入口
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) {
// 注册窗口类
WNDCLASSEX wc;
wc.cbSize = sizeof(wc);
wc.style = 0;
wc.lpfnWndProc = WndProc;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = hInstance;
wc.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszMenuName = NULL;
wc.lpszClassName = "MainWindow";
wc.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
if (!RegisterClassEx(&wc)) {
MessageBox(NULL, "窗口类注册失败!", "错误", MB_ICONERROR | MB_OK);
return 0;
}
// 创建窗口
hwnd = CreateWindowEx(0, "MainWindow", "词频统计", WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 520, 300, NULL, NULL, hInstance, NULL);
if (hwnd == NULL) {
MessageBox(NULL, "窗口创建失败!", "错误", MB_ICONERROR | MB_OK);
return 0;
}
// 显示窗口
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
```
阅读全文
相关推荐








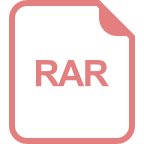





