使用c++写一个 建立一个windows对话框图形环境应用程序,添加相关编辑框和按钮,点击按钮直接运行固定txt文件(或打开文件对话框,选择 txt文件),统计文件中词的频数,并在图形界面编辑框中显示频数最大10个词,和它的频数。
时间: 2024-02-16 12:03:42 浏览: 31
好的,以下是一个C++的实现示例。这个示例使用了MFC库和STL库。
首先,你需要创建一个MFC对话框应用程序,并添加一个编辑框和一个按钮。在按钮的单击事件中,我们将处理文件读取和单词统计的逻辑。
在你的 .h 文件中,添加以下代码:
```c++
#include <map>
#include <string>
class CMyDialog : public CDialogEx
{
// ...
private:
std::map<std::string, int> m_wordCount;
CString m_filePath;
CEdit m_resultEdit;
// ...
};
```
这里我们使用了一个 STL 中的 map 容器来存储单词和相应的频率,同时还声明了一个变量来存储选择的文件路径和一个编辑框对象来显示结果。
在你的 .cpp 文件中,添加以下代码:
```c++
void CMyDialog::OnBnClickedButton1()
{
// 打开文件对话框
CFileDialog dlg(TRUE, NULL, NULL, OFN_HIDEREADONLY | OFN_OVERWRITEPROMPT, _T("Text Files(*.txt)|*.txt||"), this);
if (dlg.DoModal() == IDOK)
{
m_filePath = dlg.GetPathName();
// 打开文件
std::ifstream inFile(m_filePath);
if (!inFile.is_open())
{
MessageBox(_T("Failed to open file!"));
return;
}
// 读取文件内容
std::string word;
while (inFile >> word)
{
// 去掉标点符号和空格
word.erase(std::remove_if(word.begin(), word.end(), [](char c) { return !std::isalpha(c); }), word.end());
// 转换为小写
std::transform(word.begin(), word.end(), word.begin(), [](char c) { return std::tolower(c); });
if (word.empty()) continue;
// 统计单词频率
m_wordCount[word]++;
}
// 关闭文件
inFile.close();
// 排序并显示结果
std::vector<std::pair<std::string, int>> wordVec(m_wordCount.begin(), m_wordCount.end());
std::sort(wordVec.begin(), wordVec.end(), [](const std::pair<std::string, int>& a, const std::pair<std::string, int>& b) { return a.second > b.second; });
CString resultText;
for (int i = 0; i < 10 && i < wordVec.size(); i++)
{
CString line;
line.Format(_T("%s: %d\r\n"), CString(wordVec[i].first.c_str()), wordVec[i].second);
resultText += line;
}
m_resultEdit.SetWindowText(resultText);
}
}
```
这段代码实现了按钮的单击事件处理逻辑。首先,我们打开一个文件对话框,让用户选择要分析的txt文件。如果用户选择了一个文件,我们将读取文件内容并统计其中每个单词出现的频数。然后,我们将结果排序,并在编辑框中显示频数最高的10个单词及其频率。
这个示例代码只是一个简单的实现,你可能需要根据实际需求进行修改或扩展。同时,你也需要注意处理异常情况和错误检查。
相关推荐
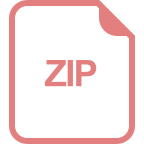
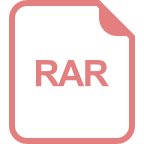
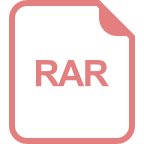














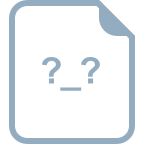