用C++建立一个windows对话框图形环境应用程序,添加相关编辑框和按钮,点击按钮直接运行固定txt文件(或打开文件对话框,选择 txt文件),统计文件中词的频数,并在图形界面编辑框中显示频数最大10个词,和它的频数。
时间: 2023-07-15 20:15:47 浏览: 100
这个任务可以分成以下几个步骤来完成:
1. 创建一个新的Windows对话框应用程序项目。
2. 在对话框上添加一个编辑框和一个按钮控件。
3. 给按钮控件添加一个响应函数,用于处理按钮点击事件。
4. 在响应函数中添加文件选择对话框,让用户选择要统计频数的txt文件。
5. 读取选定的文件中的内容,并统计每个单词出现的频数。
6. 将频数最大的前10个单词及其频数显示在编辑框中。
下面是一个简单的实现示例:
```cpp
// Step 1: 创建一个新的Windows对话框应用程序项目,并添加以下代码。
#include "stdafx.h"
#include "MyDialog.h"
#include <fstream>
#include <map>
#define MAX_WORDS 10
// Step 2: 在对话框资源中添加一个编辑框(IDC_EDIT1)和一个按钮控件(IDC_BUTTON1)。
// Step 3: 给按钮控件添加一个响应函数,用于处理按钮点击事件。
void CMyDialog::OnBnClickedButton1()
{
// Step 4: 在响应函数中添加文件选择对话框,让用户选择要统计频数的txt文件。
CString filter = _T("Text files (*.txt)|*.txt|All Files (*.*)|*.*||");
CFileDialog dlg(TRUE, NULL, NULL, OFN_HIDEREADONLY, filter);
if (dlg.DoModal() == IDOK)
{
CString filename = dlg.GetPathName();
// Step 5: 读取选定的文件中的内容,并统计每个单词出现的频数。
std::ifstream file(filename);
std::map<std::string, int> wordCount;
std::string word;
while (file >> word)
{
// 去掉单词中的标点符号和空格
word.erase(remove_if(word.begin(), word.end(), [](char c) { return ispunct(c) || isspace(c); }), word.end());
// 转换为小写字母
transform(word.begin(), word.end(), word.begin(), [](char c) { return tolower(c); });
// 更新单词频数
if (!word.empty())
{
wordCount[word]++;
}
}
// Step 6: 将频数最大的前10个单词及其频数显示在编辑框中。
std::vector<std::pair<std::string, int>> topWords;
for (auto&& pair : wordCount)
{
topWords.push_back(pair);
}
sort(topWords.begin(), topWords.end(), [](auto&& a, auto&& b) { return a.second > b.second; });
CString text;
for (int i = 0; i < min(MAX_WORDS, (int)topWords.size()); i++)
{
text.AppendFormat(_T("%S (%d)\r\n"), topWords[i].first.c_str(), topWords[i].second);
}
SetDlgItemText(IDC_EDIT1, text);
}
}
```
注意,这只是一个简单的实现,可能存在一些问题,比如无法处理一些特殊字符,以及可能会有性能问题,如果对于特别大的文件。
相关推荐
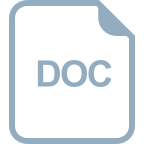
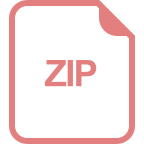
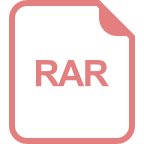
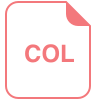













