如何用opencv-python将图片旋转
时间: 2023-08-20 21:06:09 浏览: 195
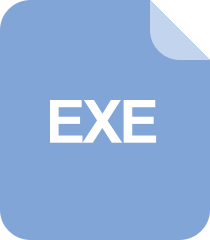
opencv实现图片旋转
可以使用OpenCV库中的函数`cv2.getRotationMatrix2D`和`cv2.warpAffine`来实现图像旋转。具体步骤如下:
1. 读入原始图像。
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
```
2. 计算旋转矩阵。
```python
(h, w) = img.shape[:2]
(center_x, center_y) = (w // 2, h // 2) # 图像中心点坐标
angle = 45 # 旋转角度
scale = 1.0 # 缩放比例
M = cv2.getRotationMatrix2D((center_x, center_y), angle, scale)
```
`cv2.getRotationMatrix2D`函数的第一个参数是旋转中心点的坐标,第二个参数是旋转角度,第三个参数是缩放比例。
3. 进行图像旋转。
```python
rotated_img = cv2.warpAffine(img, M, (w, h))
```
`cv2.warpAffine`函数的第一个参数是原始图像,第二个参数是旋转矩阵,第三个参数是输出图像的大小。
完整代码如下:
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
(h, w) = img.shape[:2]
(center_x, center_y) = (w // 2, h // 2)
angle = 45
scale = 1.0
M = cv2.getRotationMatrix2D((center_x, center_y), angle, scale)
rotated_img = cv2.warpAffine(img, M, (w, h))
cv2.imshow('Rotated Image', rotated_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
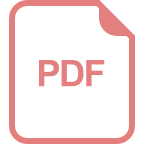
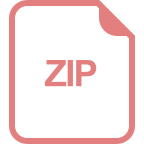
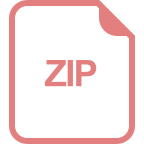
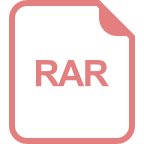
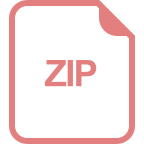


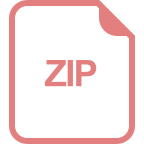
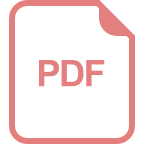
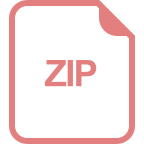
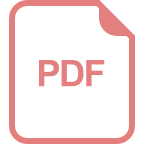
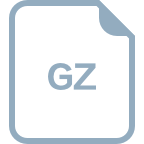

