How to coding a divider with Verilog HDL
时间: 2024-05-11 18:13:55 浏览: 115
To code a divider with Verilog HDL, you can follow these steps:
1. Define the module interface with input and output ports. The input ports should be the dividend and divisor, and the output port should be the quotient.
```
module divider (
input [31:0] dividend,
input [31:0] divisor,
output reg [31:0] quotient
);
```
2. Define any necessary internal wires and registers. In this case, we need a register to hold the remainder and a wire to hold the quotient.
```
reg [31:0] remainder;
wire [31:0] quotient;
```
3. Initialize the remainder register to the dividend value.
```
always @(*) begin
remainder <= dividend;
end
```
4. Use a loop to perform the division. The loop should iterate 32 times, since we are working with 32-bit numbers. In each iteration, shift the remainder left by 1 bit and add the next bit of the divisor. If the result is greater than or equal to the divisor, subtract the divisor from the result and set the corresponding bit of the quotient to 1. Otherwise, leave the quotient bit at 0.
```
integer i;
always @(*) begin
for (i = 31; i >= 0; i--) begin
remainder <= {remainder[30:0], 1'b0};
if (remainder >= divisor) begin
remainder <= remainder - divisor;
quotient[i] <= 1'b1;
end else begin
quotient[i] <= 1'b0;
end
end
end
```
5. Assign the quotient wire to the output port.
```
assign quotient = quotient;
```
6. End the module definition.
```
endmodule
```
阅读全文
相关推荐
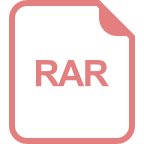
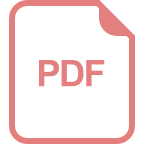
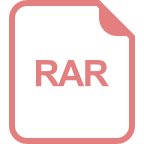















