使用vue实现一个手机端外卖饿了么app并且展示代码
时间: 2023-11-27 11:54:51 浏览: 161
这是一个比较大的项目,涉及到很多知识点,包括但不限于Vue框架、Vue Router、Vuex、Axios、ElementUI等。以下是一个简单的实现外卖饿了么app的示例代码:
App.vue
```
<template>
<div id="app">
<router-view></router-view>
</div>
</template>
<script>
export default {
name: "App",
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
}
</style>
```
router.js
```
import Vue from "vue";
import Router from "vue-router";
import Home from "./views/Home.vue";
import Cart from "./views/Cart.vue";
import Profile from "./views/Profile.vue";
import Search from "./views/Search.vue";
Vue.use(Router);
export default new Router({
routes: [
{
path: "/",
name: "home",
component: Home,
},
{
path: "/cart",
name: "cart",
component: Cart,
},
{
path: "/profile",
name: "profile",
component: Profile,
},
{
path: "/search",
name: "search",
component: Search,
},
],
});
```
Home.vue
```
<template>
<div class="home">
<h1>Home Page</h1>
</div>
</template>
<script>
export default {
name: "Home",
};
</script>
<style>
.home {
padding: 20px;
}
</style>
```
Cart.vue
```
<template>
<div class="cart">
<h1>Cart Page</h1>
</div>
</template>
<script>
export default {
name: "Cart",
};
</script>
<style>
.cart {
padding: 20px;
}
</style>
```
Profile.vue
```
<template>
<div class="profile">
<h1>Profile Page</h1>
</div>
</template>
<script>
export default {
name: "Profile",
};
</script>
<style>
.profile {
padding: 20px;
}
</style>
```
Search.vue
```
<template>
<div class="search">
<h1>Search Page</h1>
</div>
</template>
<script>
export default {
name: "Search",
};
</script>
<style>
.search {
padding: 20px;
}
</style>
```
以上代码只是一个简单的示例,还需要自行添加代码实现功能,比如登录、注册、商品列表、购物车等。同时,还需要使用Mock数据模拟后端接口数据,使用Axios库进行数据请求。如果需要实现更多功能,还需要使用Vuex进行状态管理。
阅读全文
相关推荐
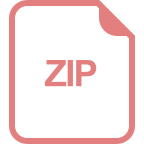
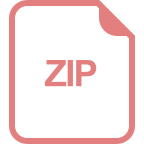
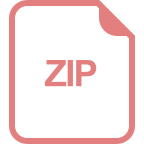
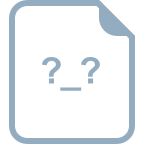
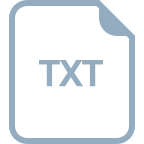
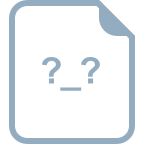
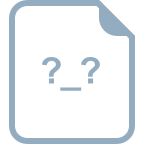
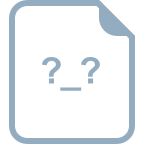
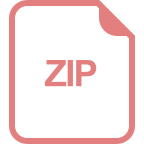
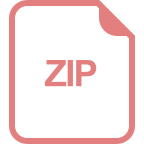
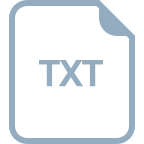
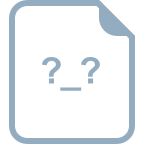
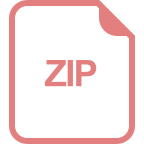
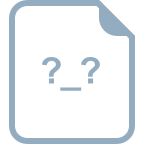
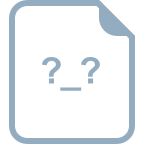