在C# 中convert
时间: 2024-10-10 11:15:43 浏览: 38
在C#中,`Convert`类提供了一系列静态方法,用于在.NET框架的不同数据类型之间进行基本类型的转换。这些方法包括但不限于以下几个常见的用途:
1. `Convert.ToInt32()` 和 `Convert.ToInt64()`:这两个方法分别用于将给定的对象转换为32位和64位整数(Int32和Int64)。它们类似于`int.Parse()`,但如果输入不是有效的数字,`Convert`方法会返回默认值(对于整数通常是0)而不会抛出异常。
2. `Convert.ToBoolean()` 和 `Convert.ToInt16()` 等:同样地,有对应的方法可以将字符串、枚举等转换为布尔值和16位整数等其他基本类型。
3. `Convert.ToDateTime()`:将字符串或其他格式的日期时间字符串转换为DateTime对象。
4. `Convert.ToDecimal()` 和 `Convert.ToDouble()`:用于将值从一种浮点数类型转换到另一种,如Decimal和Double。
5. `Convert.FromBase64String()` 和 `Convert.ToBase64String()`:用于字符串之间的二进制编码和解码操作。
例子:
```csharp
string strNumber = "123";
int integerValue = Convert.ToInt32(strNumber);
double doubleValue = Convert.ToDouble(integerValue);
```
相关问题
c#数据convert类型转换
C# 提供了一个 `Convert` 类,它位于 `System` 命名空间中,用于数据类型的转换。`Convert` 类提供了一组静态方法,可以将一种数据类型转换为另一种数据类型。这种转换可以是显式的也可以是隐式的,但大多数情况下需要显式调用。以下是 `Convert` 类中一些常用方法的示例:
1. `Convert.ToInt32()`:将其他数据类型转换为32位整数。
2. `Convert.ToString()`:将其他数据类型转换为字符串。
3. `Convert.ToDouble()`:将其他数据类型转换为双精度浮点数。
4. `Convert.ToSingle()`:将其他数据类型转换为单精度浮点数。
5. `Convert.ToBoolean()`:将其他数据类型转换为布尔值。
6. `Convert.ToDateTime()`:将其他数据类型转换为日期时间。
7. `Convert.ToChar()`:将其他数据类型转换为字符。
转换时需要注意,`Convert` 类的方法在无法进行转换时会抛出异常,如 `OverflowException` 或 `FormatException`。因此,在使用 `Convert` 类进行转换时,应该确保源数据类型可以被安全地转换为目标类型。
例如,将字符串转换为整数的代码如下:
```csharp
string strValue = "100";
int intValue = Convert.ToInt32(strValue);
```
在使用 `Convert` 类进行类型转换时,最好先检查数据类型或使用异常处理机制来捕获可能发生的转换错误。
c# mongodb convert between model and bson
To convert between a model and BSON in C# with MongoDB, you can use the `BsonDocument` class and the `BsonSerializer` class from the MongoDB C# driver.
To convert a model to BSON:
```
using MongoDB.Bson;
using MongoDB.Bson.Serialization;
public class Person
{
public ObjectId Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
}
Person person = new Person { Name = "John", Age = 30 };
BsonDocument bsonDocument = person.ToBsonDocument();
```
To convert BSON to a model:
```
using MongoDB.Bson;
using MongoDB.Bson.Serialization;
BsonDocument bsonDocument = ... // retrieve the document from MongoDB
Person person = BsonSerializer.Deserialize<Person>(bsonDocument);
```
Note that you should replace the `...` with the code that retrieves the document from MongoDB.
If you need to convert a list of models to BSON, you can use `BsonArray` and `ToBsonDocumentArray`:
```
List<Person> people = new List<Person>
{
new Person { Name = "John", Age = 30 },
new Person { Name = "Jane", Age = 25 }
};
BsonArray bsonArray = new BsonArray(people.Select(x => x.ToBsonDocument()));
BsonDocument bsonDocument = new BsonDocument { { "people", bsonArray } };
```
And to convert BSON to a list of models:
```
BsonDocument bsonDocument = ... // retrieve the document from MongoDB
BsonArray bsonArray = bsonDocument["people"].AsBsonArray;
List<Person> people = bsonArray.Select(x => BsonSerializer.Deserialize<Person>(x.AsBsonDocument)).ToList();
```
Again, you should replace the `...` with the code that retrieves the document from MongoDB.
阅读全文
相关推荐
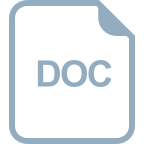
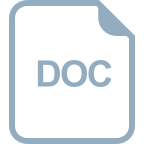
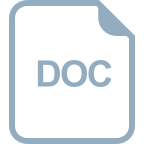
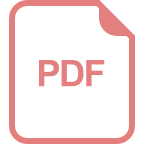
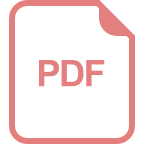
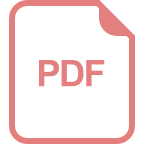
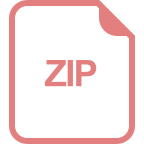
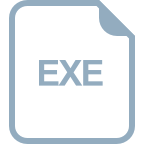
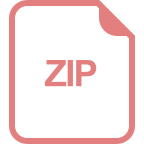
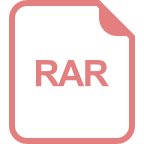
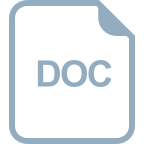





