antdtable可编辑表格
时间: 2024-06-19 18:02:43 浏览: 11
antdtable是一个基于React和Ant Design的表格组件,它提供了一系列易于使用的API,可以让用户轻松地创建出高度定制化的表格。其中,可编辑表格是antdtable中的一个重要特性,可以让用户直接在表格中进行数据编辑,提高了数据录入的效率。
antdtable可编辑表格的主要特点如下:
1. 支持行内编辑和弹出框编辑两种方式。
2. 可以通过设置不同的组件类型,例如Input、Select等,来实现不同类型的数据输入。
3. 提供了onChange、onSave、onCancel等事件回调函数,可以在数据发生变化时进行相应处理。
4. 可以通过设置rules属性,来实现对表格数据的校验和错误提示。
如果您需要了解更多关于antdtable可编辑表格的使用方法和API,请参考Ant Design官方文档:https://ant.design/components/table-cn/#components-table-demo-edit-row。
相关问题
antd可编辑表格和拖拽
Antd提供了Table组件,可以实现表格的展示和编辑功能。而要实现可编辑表格和拖拽功能,需要对Table组件进行一些扩展。
1. 可编辑表格
Antd的Table组件提供了editable属性,开启该属性后,表格单元格就可以进行编辑了。同时,还需要在columns中配置每一列是否可编辑,以及编辑时的类型和属性。
例如,下面的代码可以实现一个简单的可编辑表格:
```jsx
import { Table, Input, InputNumber, Popconfirm, Form } from 'antd';
import React, { useState } from 'react';
const EditableCell = ({
editing,
dataIndex,
title,
inputType,
record,
index,
children,
...restProps
}) => {
const inputNode = inputType === 'number' ? <InputNumber /> : <Input />;
return (
<td {...restProps}>
{editing ? (
<Form.Item
name={dataIndex}
style={{
margin: 0,
}}
rules={[
{
required: true,
message: `Please Input ${title}!`,
},
]}
>
{inputNode}
</Form.Item>
) : (
children
)}
</td>
);
};
const EditableTable = () => {
const [form] = Form.useForm();
const [data, setData] = useState([
{
key: '1',
name: 'Edward King 0',
age: 32,
address: 'London, Park Lane no. 0',
},
{
key: '2',
name: 'Edward King 1',
age: 32,
address: 'London, Park Lane no. 1',
},
]);
const [editingKey, setEditingKey] = useState('');
const isEditing = (record) => record.key === editingKey;
const edit = (record) => {
form.setFieldsValue({
name: '',
age: '',
address: '',
...record,
});
setEditingKey(record.key);
};
const cancel = () => {
setEditingKey('');
};
const save = async (key) => {
try {
const row = await form.validateFields();
const newData = [...data];
const index = newData.findIndex((item) => key === item.key);
if (index > -1) {
const item = newData[index];
newData.splice(index, 1, { ...item, ...row });
setData(newData);
setEditingKey('');
} else {
newData.push(row);
setData(newData);
setEditingKey('');
}
} catch (errInfo) {
console.log('Validate Failed:', errInfo);
}
};
const columns = [
{
title: 'Name',
dataIndex: 'name',
width: '25%',
editable: true,
},
{
title: 'Age',
dataIndex: 'age',
width: '15%',
editable: true,
},
{
title: 'Address',
dataIndex: 'address',
width: '40%',
editable: true,
},
{
title: 'Operation',
dataIndex: 'operation',
render: (_, record) => {
const editable = isEditing(record);
return editable ? (
<span>
<a
href="javascript:;"
onClick={() => save(record.key)}
style={{
marginRight: 8,
}}
>
Save
</a>
<Popconfirm title="Sure to cancel?" onConfirm={cancel}>
<a>Cancel</a>
</Popconfirm>
</span>
) : (
<a disabled={editingKey !== ''} onClick={() => edit(record)}>
Edit
</a>
);
},
},
];
const mergedColumns = columns.map((col) => {
if (!col.editable) {
return col;
}
return {
...col,
onCell: (record) => ({
record,
inputType: col.dataIndex === 'age' ? 'number' : 'text',
dataIndex: col.dataIndex,
title: col.title,
editing: isEditing(record),
}),
};
});
return (
<Form form={form} component={false}>
<Table
components={{
body: {
cell: EditableCell,
},
}}
bordered
dataSource={data}
columns={mergedColumns}
rowClassName="editable-row"
pagination={{
onChange: cancel,
}}
/>
</Form>
);
};
export default EditableTable;
```
2. 拖拽
Antd的Table组件默认不支持拖拽功能,但是可以通过一些插件来实现。
其中,react-beautiful-dnd是一个比较常用的拖拽插件,可以很方便地和Antd的Table组件结合使用。具体实现步骤如下:
1. 安装react-beautiful-dnd插件
```bash
npm install react-beautiful-dnd
```
2. 将Table组件封装成DragDropContext组件
```jsx
import { Table } from 'antd';
import { DragDropContext, Droppable, Draggable } from 'react-beautiful-dnd';
const DragTable = ({ columns, dataSource, onDragEnd }) => {
return (
<DragDropContext onDragEnd={onDragEnd}>
<Droppable droppableId="droppable">
{(provided) => (
<Table
{...provided.droppableProps}
ref={provided.innerRef}
columns={columns}
dataSource={dataSource}
pagination={false}
rowClassName="drag-row"
onRow={(record, index) => ({
index,
moveRow: true,
})}
/>
)}
</Droppable>
</DragDropContext>
);
};
export default DragTable;
```
3. 实现onDragEnd回调函数
```jsx
const onDragEnd = (result) => {
if (!result.destination) {
return;
}
const { source, destination } = result;
// 根据拖拽后的顺序重新排序dataSource
const newData = [...dataSource];
const [removed] = newData.splice(source.index, 1);
newData.splice(destination.index, 0, removed);
// 更新dataSource
setData(newData);
};
```
最后,在页面中引用DragTable组件即可实现拖拽功能。例如:
```jsx
<DragTable columns={columns} dataSource={data} onDragEnd={onDragEnd} />
```
antdpro的可编辑表格
Ant Design Pro 提供了一个可编辑表格的组件,名为 EditableTable,可以轻松地实现表格数据的编辑和保存。下面是一个简单的例子:
```jsx
import { useState } from 'react';
import { Button, Table, Input } from 'antd';
const EditableTable = () => {
const [data, setData] = useState([
{ id: 1, name: 'John', age: 24 },
{ id: 2, name: 'Mary', age: 32 },
{ id: 3, name: 'Bob', age: 45 },
]);
const handleSave = (row) => {
const newData = [...data];
const index = newData.findIndex((item) => row.id === item.id);
newData[index] = row;
setData(newData);
};
const columns = [
{
title: 'Name',
dataIndex: 'name',
editable: true,
},
{
title: 'Age',
dataIndex: 'age',
editable: true,
},
{
title: 'Action',
render: (_, row) => (
<Button type="primary" onClick={() => handleSave(row)}>
Save
</Button>
),
},
];
const components = {
body: {
row: EditableRow,
cell: EditableCell,
},
};
const EditableRow = ({ index, ...restProps }) => (
<Table.Row {...restProps} />
);
const EditableCell = ({
title,
editable,
children,
dataIndex,
record,
handleSave,
...restProps
}) => {
const [editing, setEditing] = useState(false);
const [inputValue, setInputValue] = useState(children);
const toggleEdit = () => {
setEditing(!editing);
setInputValue(record[dataIndex]);
};
const handleChange = (event) => {
setInputValue(event.target.value);
};
const handleBlur = () => {
setEditing(false);
handleSave({ ...record, [dataIndex]: inputValue });
};
return (
<td {...restProps}>
{editable ? (
editing ? (
<Input value={inputValue} onChange={handleChange} onBlur={handleBlur} />
) : (
<div style={{ paddingRight: 24 }} onClick={toggleEdit}>
{children}
</div>
)
) : (
children
)}
</td>
);
};
return (
<Table
bordered
dataSource={data}
columns={columns}
components={components}
rowKey="id"
/>
);
};
export default EditableTable;
```
注意,这个例子中使用了 antd 的 Input 和 Button 组件,如果没有安装 antd,需要先安装才能运行。另外,这个例子还使用了 React Hooks 实现了组件的状态管理。
相关推荐
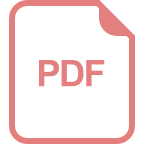
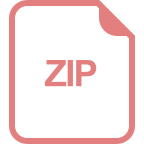
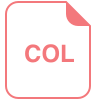
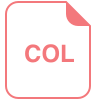
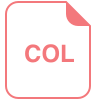
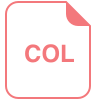
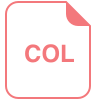







