java lastIndexOf() 代码示例
时间: 2023-09-09 21:05:19 浏览: 48
### 回答1:
当一个字符串S中包含多个相同的子字符串时,lastIndexOf() 方法返回该子字符串最后出现的位置。例如:
String s = "I have a cat, and she has a cat too.";
int lastIndex = s.lastIndexOf("cat");
System.out.println(lastIndex); // 输出 31
这段代码会输出字符串 "cat" 最后出现的位置,也就是第二个 "cat" 出现的位置,即索引 31。如果该子字符串没有出现过,lastIndexOf() 方法会返回 -1。
### 回答2:
lastIndexOf() 是Java中的String类的方法之一,它用于返回指定字符或子字符串在字符串中最后一次出现的位置。它的函数签名如下所示:
int lastIndexOf(String str)
其中,str是要搜索的字符或子字符串。该方法从字符串的末尾开始向前搜索,返回找到的最后一个匹配项在字符串中的索引。如果找不到匹配项,则返回-1。
以下是一个代码示例:
```java
public class LastIndexOfExample {
public static void main(String[] args) {
String str = "Hello World!";
int lastIndex1 = str.lastIndexOf('o');
System.out.println("最后一个'o'的索引位置为:" + lastIndex1);
int lastIndex2 = str.lastIndexOf("World");
System.out.println("最后一次出现'World'的索引位置为:" + lastIndex2);
int lastIndex3 = str.lastIndexOf("o", 6);
System.out.println("从索引6开始倒序搜索,最后一个'o'的索引位置为:" + lastIndex3);
int lastIndex4 = str.lastIndexOf("abc");
System.out.println("找不到'abc',返回索引-1:" + lastIndex4);
}
}
```
输出结果为:
```
最后一个'o'的索引位置为:7
最后一次出现'World'的索引位置为:6
从索引6开始倒序搜索,最后一个'o'的索引位置为:4
找不到'abc',返回索引-1:-1
```
在示例中,我们首先使用lastIndexOf()方法找到字符串中最后一个字符'o'的索引位置,然后找到子字符串"World"和一个字段"o"在字符串中最后一次出现的索引位置。最后,我们尝试搜索一个不存在于字符串中的子字符串"abc",返回-1表示找不到。
### 回答3:
lastIndexOf()方法是在Java字符串中用于返回指定字符或子字符串最后一次出现的位置。它可以用于判断字符或子字符串在字符串中的位置,并返回最后一次出现的索引位置。
下面是一个关于lastIndexOf()方法的示例代码:
```java
public class LastIndexOfExample {
public static void main(String[] args) {
String str = "Hello World and World";
// 找到'W'最后一次出现的位置
int lastIndex = str.lastIndexOf('W');
System.out.println("最后一个'W'的位置: " + lastIndex);
// 找到'and'最后一次出现的位置
lastIndex = str.lastIndexOf("and");
System.out.println("最后一个'and'的位置: " + lastIndex);
// 找到'earth'最后一次出现的位置(不存在)
lastIndex = str.lastIndexOf("earth");
System.out.println("最后一个'earth'的位置: " + lastIndex);
}
}
```
在上述示例中,定义了一个字符串变量str,然后使用lastIndexOf()方法来查找字符串中某个字符或子字符串最后一次出现的位置。
通过使用单个字符作为参数,例如'W',可以找到该字符最后一次出现的索引位置。在示例中,'W'最后一次出现的位置是12。
通过使用字符串作为参数,例如"and",可以找到该子字符串最后一次出现的索引位置。在示例中,"and"最后一次出现的位置是11。
如果查询的字符或子字符串不存在于原始字符串中,则返回-1。在示例中,"earth"在原始字符串中不存在,因此返回-1。
通过使用lastIndexOf()方法,您可以方便地找到字符或子字符串在字符串中的最后一次出现的位置。
相关推荐
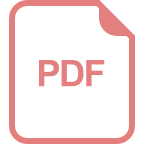
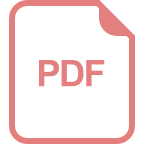
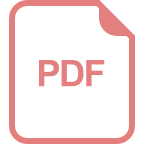














