多线程下载文件及进度条java代码示例
时间: 2024-02-04 16:04:01 浏览: 118
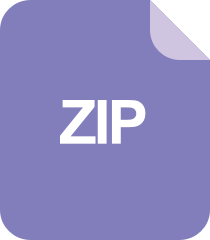
java 多线程下载实例

以下是一个简单的多线程下载文件并显示进度条的Java代码示例:
```java
import java.io.BufferedInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.URL;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class MultiThreadedDownloader {
private static final int BUFFER_SIZE = 4096;
public static void main(String[] args) {
String fileUrl = "http://example.com/file.zip";
String saveDir = "download";
try {
downloadFile(fileUrl, saveDir);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void downloadFile(String fileUrl, String saveDir) throws IOException {
URL url = new URL(fileUrl);
String fileName = fileUrl.substring(fileUrl.lastIndexOf("/") + 1);
BufferedInputStream in = new BufferedInputStream(url.openStream());
FileOutputStream fos = new FileOutputStream(saveDir + "/" + fileName);
ExecutorService executorService = Executors.newFixedThreadPool(5); // 设置线程池大小为5
byte[] buffer = new byte[BUFFER_SIZE];
int bytesRead;
long downloadedBytes = 0;
long fileSize = url.openConnection().getContentLength();
while ((bytesRead = in.read(buffer, 0, BUFFER_SIZE)) != -1) {
executorService.execute(new DownloadWorker(buffer, bytesRead, fos));
downloadedBytes += bytesRead;
printProgress(downloadedBytes, fileSize);
}
in.close();
fos.close();
executorService.shutdown();
}
private static void printProgress(long downloadedBytes, long fileSize) {
int percent = (int) ((downloadedBytes * 100) / fileSize);
System.out.print("\rDownloaded: " + percent + "%");
}
private static class DownloadWorker implements Runnable {
private byte[] buffer;
private int bytesRead;
private FileOutputStream fos;
public DownloadWorker(byte[] buffer, int bytesRead, FileOutputStream fos) {
this.buffer = buffer;
this.bytesRead = bytesRead;
this.fos = fos;
}
@Override
public void run() {
try {
fos.write(buffer, 0, bytesRead);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
这个示例代码使用了Java的多线程和线程池来实现多线程下载文件。它会创建一个固定大小为5的线程池,每个线程负责读取缓冲区中的数据,并将数据写入文件中。同时,它会计算下载进度并显示在控制台上。
阅读全文
相关推荐
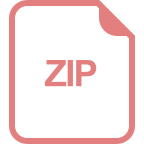
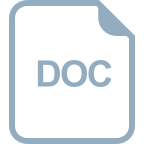
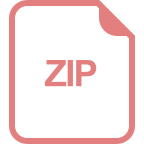
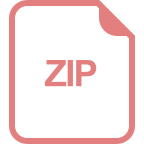
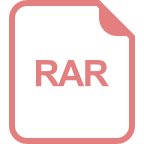
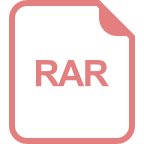
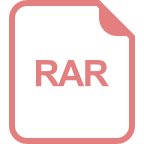
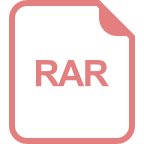
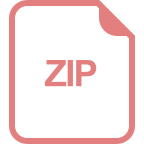
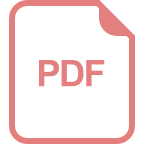
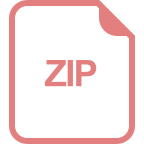
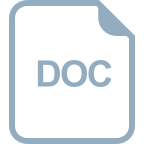
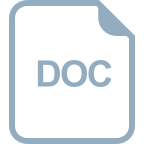
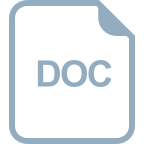
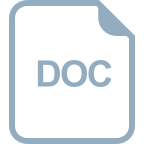
