多線程計算卷积c++代碼
时间: 2023-10-18 15:07:12 浏览: 20
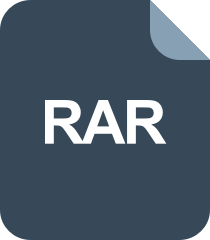
C++实现卷积码

以下是一個基本的多線程計算卷積的代碼,使用OpenMP庫實現。該代碼使用了一個矩陣乘法的方法來計算卷積,並且使用了多線程來加速計算。
```
#include <iostream>
#include <omp.h>
using namespace std;
void convolve(int* input, int* kernel, int* output, int input_width, int input_height, int kernel_width, int kernel_height)
{
int output_width = input_width - kernel_width + 1;
int output_height = input_height - kernel_height + 1;
#pragma omp parallel for
for(int i = 0; i < output_width; i++)
{
for(int j = 0; j < output_height; j++)
{
int sum = 0;
for(int k = 0; k < kernel_width; k++)
{
for(int l = 0; l < kernel_height; l++)
{
int input_index = (i + k) * input_height + (j + l);
int kernel_index = k * kernel_height + l;
sum += input[input_index] * kernel[kernel_index];
}
}
int output_index = i * output_height + j;
output[output_index] = sum;
}
}
}
int main()
{
// Initialize input and kernel matrices
int input_width = 10;
int input_height = 10;
int kernel_width = 3;
int kernel_height = 3;
int input[input_width * input_height];
int kernel[kernel_width * kernel_height];
int output[(input_width - kernel_width + 1) * (input_height - kernel_height + 1)];
for(int i = 0; i < input_width * input_height; i++)
{
input[i] = rand() % 10;
}
for(int i = 0; i < kernel_width * kernel_height; i++)
{
kernel[i] = rand() % 10;
}
// Calculate convolution using multiple threads
double start_time = omp_get_wtime();
convolve(input, kernel, output, input_width, input_height, kernel_width, kernel_height);
double end_time = omp_get_wtime();
// Print output
cout << "Input Matrix:" << endl;
for(int i = 0; i < input_width; i++)
{
for(int j = 0; j < input_height; j++)
{
cout << input[i * input_height + j] << "\t";
}
cout << endl;
}
cout << endl << "Kernel Matrix:" << endl;
for(int i = 0; i < kernel_width; i++)
{
for(int j = 0; j < kernel_height; j++)
{
cout << kernel[i * kernel_height + j] << "\t";
}
cout << endl;
}
cout << endl << "Output Matrix:" << endl;
for(int i = 0; i < (input_width - kernel_width + 1); i++)
{
for(int j = 0; j < (input_height - kernel_height + 1); j++)
{
cout << output[i * (input_height - kernel_height + 1) + j] << "\t";
}
cout << endl;
}
cout << endl << "Execution time: " << end_time - start_time << " seconds" << endl;
return 0;
}
```
阅读全文
相关推荐




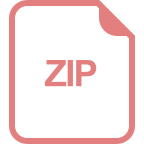
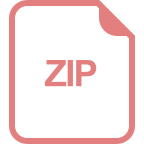
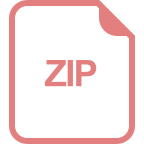
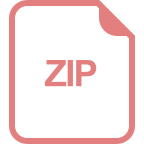
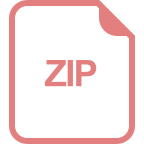
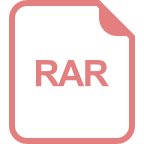
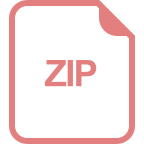
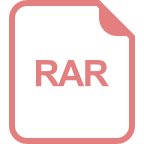
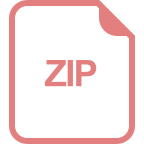
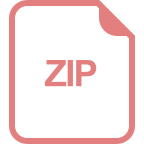
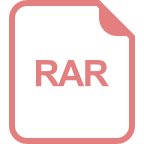
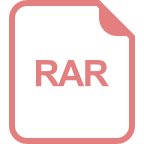