用python写一个手机号码归属地的判断
时间: 2023-05-26 22:05:18 浏览: 123
使用Python可以轻松实现手机号码归属地的判断。可以通过访问第三方API或者本地的号码库来实现。
1. 通过访问第三方API
可以使用聚合数据、阿里云等第三方提供的手机号码归属地API,通过获取接口返回的数据来实现。
首先需要注册相应的服务,获取API的访问密钥。假设获取到的密钥为KEY。代码实现如下:
```python
import requests
phone_number = input("请输入手机号码:")
url = "http://apis.juhe.cn/mobile/get?phone=" + phone_number + "&key=" + KEY
response = requests.get(url)
result = response.json()
if result["error_code"] == 0:
province = result["result"]["province"]
city = result["result"]["city"]
print("该手机号码归属地为:{} {}".format(province, city))
else:
print("查询失败:" + result["reason"])
```
2. 通过本地号码库实现
可以使用开源的号码归属地数据库,如ipip.net提供的免费版本号码库。将下载的归属地数据库文件(如“17monipdb.dat”)放置项目目录下。
代码实现如下:
```python
import os
import re
import socket
def load_dat(file_path):
if not os.path.exists(file_path):
raise ValueError("The file {} doesn't exist".format(file_path))
with open(file_path, "rb") as f:
return f.read()
def find_phone_location(phone_number, db):
if not phone_number.isdigit():
raise ValueError("The provided phone number {} is not valid".format(phone_number))
if len(phone_number) < 7:
raise ValueError("The provided phone number {} is too short".format(phone_number))
phone_prefix = phone_number[0:7]
offset = int(phone_prefix[0:3])
index_offset = int(phone_prefix[3:])
offset = offset * 4
index_offset = index_offset * 4
max_comp_len = len(db) - 262144 - 4
while offset < max_comp_len:
temp = db[offset:offset+4]
st = int.from_bytes(temp, byteorder='big')
temp = db[st:st+4]
ed = int.from_bytes(temp, byteorder='big')
if index_offset < ed:
temp = db[st+4:st+7]
province_index = int.from_bytes(temp, byteorder='big')
temp = db[province_index:province_index+3]
province = temp.decode('utf-8')
temp = db[province_index+3:]
city_index = int.from_bytes(temp, byteorder='big')
temp = db[city_index:city_index+3]
city = temp.decode('utf-8')
return province, city
offset += 4
raise ValueError("The phone number {} wasn't found in the database".format(phone_number))
if __name__ == '__main__':
phone_number = input('请输入手机号码:')
db = load_dat('./17monipdb.dat')
province, city = find_phone_location(phone_number, db)
print('该手机号码归属地为:{} {}'.format(province, city))
```
阅读全文
相关推荐
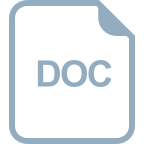
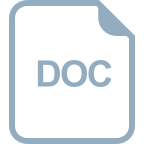
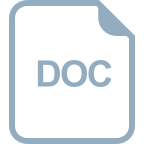

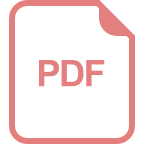
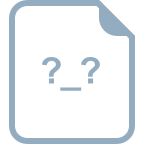
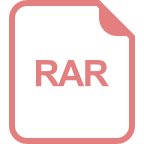
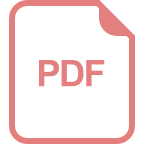
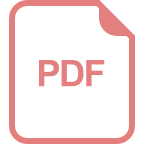
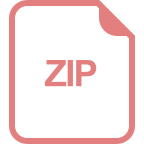
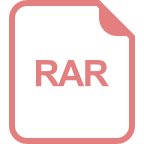
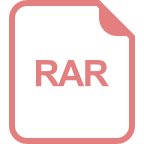
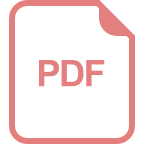
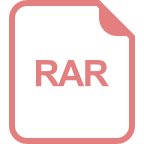
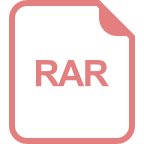
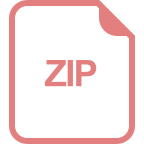
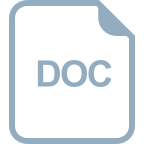
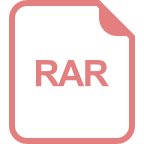