export default { name: 'HomeHeader', computed: { ...mapState(['city']) } }
时间: 2024-06-04 19:06:07 浏览: 90
这段代码是一个 Vue.js 组件的定义,其中:
- `name: 'HomeHeader'` 定义了组件的名称为 `HomeHeader`;
- `computed` 是 Vue.js 中的一个计算属性,用于根据已有的 state 数据计算出新的数据。在这里,`mapState` 函数是 Vuex 中的一个辅助函数,用于将 store 中的 state 映射到当前组件的 computed 属性中。`['city']` 表示将 `state.city` 映射为 `this.city`;
- 最后通过 `export default` 导出组件,以便在其他地方引用。
相关问题
<script> import Bscroll from 'better-scroll' import { mapMutations } from 'vuex' export default { name: 'CitySearch', props: { cities: Object }, data () { return { keyword: '', list: [], timer: null } }, computed: { hasNoData () { return !this.list.length } }, watch: { keyword () { if (this.timer) { clearTimeout(this.timer) } if (!this.keyword) { this.list = [] return }1 this.timer = setTimeout(() => { const result = [] for (let i in this.cities) { this.cities[i].forEach((value) => { if (value.spell.indexOf(this.keyword) > -1 || value.name.indexOf(this.keyword) > -1) { result.push(value) } }) } this.list = result }, 100) } }, methods: { handleCityClick (city) { this.changeCity(city) this.$router.push('/') }, ...mapMutations(['changeCity']) }, mounted () { this.scroll = new Bscroll(this.$refs.search, { click: true }) } } </script>解释一下这段代码
这是一个 Vue 组件,主要功能是实现城市搜索。下面是详细解释:
1. 引入了一个名为 `Bscroll` 的第三方滚动库。
2. 引入了 `mapMutations` 方法,用于将 Vuex 的 `changeCity` 方法映射到组件中。
3. 定义了组件的名称为 `CitySearch`,传入了一个名为 `cities` 的 props,用于接收城市数据。
4. 定义了组件的数据对象 `data`,包含了搜索关键词 `keyword`,搜索结果列表 `list`,以及一个定时器 `timer`。
5. 定义了计算属性 `hasNoData`,用于判断搜索结果列表是否为空。
6. 定义了一个监控 `keyword` 变化的 `watch`,在 `keyword` 变化时根据城市数据进行搜索,并将结果保存到 `list` 中。
7. 定义了一个 `handleCityClick` 方法,用于处理点击城市事件,将选择的城市信息传递给 `changeCity` 方法,并跳转到首页。
8. 使用 `mapMutations` 方法将 `changeCity` 方法映射到组件中。
9. 在组件的 `mounted` 钩子函数中,初始化了一个滚动条实例并绑定到组件的 `$refs.search` 上。
elementui table 使用插槽 将数组中对象的id 转为显示name
可以使用`scoped slot`和`$attrs`来实现将数组中对象的id转为显示name的功能。
首先,在定义表格列时,需要将显示id的列定义为一个 scoped slot,例如:
```html
<template>
<el-table :data="tableData" style="width: 100%">
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column label="城市">
<template slot-scope="scope">
{{ getCityName(scope.row.cityId) }}
</template>
</el-table-column>
</el-table>
</template>
```
在这个例子中,第二列使用了 scoped slot,并且使用 `getCityName` 方法将 cityId 转为了对应的城市名称。
接下来,需要在组件中定义 `getCityName` 方法,例如:
```javascript
export default {
data() {
return {
cities: [
{ id: 1, name: '北京' },
{ id: 2, name: '上海' },
{ id: 3, name: '广州' },
{ id: 4, name: '深圳' },
],
tableData: [
{ name: '张三', cityId: 1 },
{ name: '李四', cityId: 2 },
{ name: '王五', cityId: 3 },
{ name: '赵六', cityId: 4 },
],
};
},
methods: {
getCityName(cityId) {
const city = this.cities.find((c) => c.id === cityId);
return city ? city.name : '';
},
},
};
```
在这个例子中,组件的 data 中定义了一个 cities 数组,用于存储城市 id 和名称的对应关系。在 `getCityName` 方法中,根据传入的 cityId 参数,使用 Array.find 方法查找对应的城市对象,并返回城市名称。
最后,需要将 cities 数组传递给组件,可以使用 `$attrs` 来实现:
```html
<template>
<el-table :data="tableData" style="width: 100%" :attrs="attrs">
<!-- ... -->
</el-table>
</template>
<script>
export default {
data() {
return {
cities: [
{ id: 1, name: '北京' },
{ id: 2, name: '上海' },
{ id: 3, name: '广州' },
{ id: 4, name: '深圳' },
],
tableData: [
{ name: '张三', cityId: 1 },
{ name: '李四', cityId: 2 },
{ name: '王五', cityId: 3 },
{ name: '赵六', cityId: 4 },
],
};
},
methods: {
getCityName(cityId) {
const city = this.cities.find((c) => c.id === cityId);
return city ? city.name : '';
},
},
computed: {
attrs() {
return {
cities: this.cities,
};
},
},
};
</script>
```
在这个例子中,将 cities 数组通过计算属性的方式传递给了表格组件。在表格组件中,可以通过 `$attrs` 访问到这个数据,例如在 scoped slot 中使用:
```html
<template>
<el-table :data="tableData" style="width: 100%" :attrs="attrs">
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column label="城市">
<template slot-scope="scope">
{{ $attrs.cities.find((city) => city.id === scope.row.cityId)?.name }}
</template>
</el-table-column>
</el-table>
</template>
```
在这个例子中,使用了 `$attrs.cities.find` 方法来查找对应的城市对象,并使用 `?.name` 来获取城市名称。需要注意的是,这里使用了可选链操作符,需要确保代码运行的环境支持这个特性。如果不支持可选链操作符,可以使用类似 `|| ''` 的方式来避免异常。
阅读全文
相关推荐
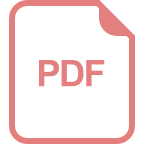
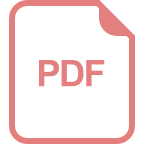
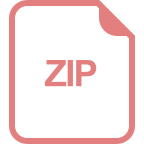
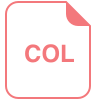
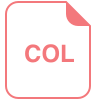



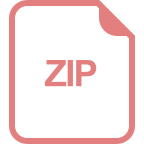
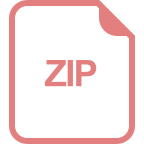
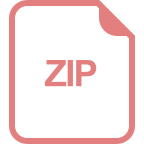
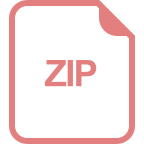