angularjs select
时间: 2023-08-18 19:14:44 浏览: 51
AngularJS provides a directive called "ng-options" to create select dropdowns. Here is an example of how to use it:
HTML code:
```html
<select ng-model="Item" ng-options="item as item.label for in items"></select>
```
In this example, the "ng-model" directive binds the selected value to a variable called "selectedItem". The "ng-options" directive is used to populate the select dropdown with options.
In your controller, you will need to define the "items" array that contains the options for the select dropdown. Each item in the array should have a "label" property, which is displayed as the option label, and optionally a "value" property, which is the selected value.
JavaScript code:
```javascript
angular.module('myApp', [])
.controller('myController', function($scope) {
$scope.items = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Option 3', value: 'option3' }
];
});
```
Make sure to include the "ng-app" and "ng-controller" directives appropriately in your HTML code to bind the controller to the view.
This is a basic example of using AngularJS to create a select dropdown. You can customize it further based on your specific requirements.
相关推荐
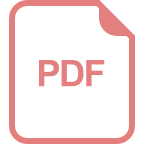
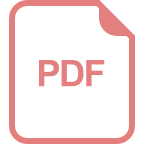
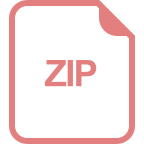
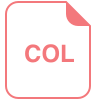













