用java写一段具有注释的代码,能够将本地文件上传到linux服务器
时间: 2023-06-14 11:07:49 浏览: 59
下面是一段用Java实现的将本地文件上传到Linux服务器的代码,注释已经添加在代码中:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStream;
import java.util.Properties;
import com.jcraft.jsch.Channel;
import com.jcraft.jsch.ChannelExec;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.Session;
public class FileUploader {
public static void main(String[] args) {
// 读取配置文件
Properties props = new Properties();
try {
props.load(new FileInputStream("config.properties"));
} catch (Exception e) {
e.printStackTrace();
}
// 连接SSH服务器
JSch jsch = new JSch();
Session session = null;
try {
session = jsch.getSession(props.getProperty("username"), props.getProperty("host"), Integer.parseInt(props.getProperty("port")));
session.setConfig("StrictHostKeyChecking", "no");
session.setPassword(props.getProperty("password"));
session.connect();
} catch (Exception e) {
e.printStackTrace();
}
// 上传文件
Channel channel = null;
InputStream in = null;
try {
channel = session.openChannel("exec");
((ChannelExec) channel).setCommand("mkdir -p " + props.getProperty("remotePath"));
channel.connect();
channel.disconnect();
channel = session.openChannel("sftp");
channel.connect();
((ChannelExec) channel).setCommand("put " + props.getProperty("localFile") + " " + props.getProperty("remotePath"));
in = channel.getInputStream();
channel.connect();
byte[] tmp = new byte[1024];
while (true) {
while (in.available() > 0) {
int i = in.read(tmp, 0, 1024);
if (i < 0)
break;
System.out.print(new String(tmp, 0, i));
}
if (channel.isClosed()) {
if (in.available() > 0)
continue;
System.out.println("upload file success!");
break;
}
try {
Thread.sleep(1000);
} catch (Exception ee) {
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
in.close();
} catch (Exception e) {
}
channel.disconnect();
session.disconnect();
}
}
}
```
这段代码通过使用JSch库实现了SSH连接和SFTP上传文件的功能,其中需要在配置文件中指定用户名、密码、主机名、端口号、本地文件路径和远程服务器文件路径等参数。
相关推荐
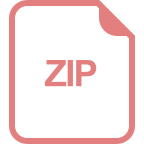
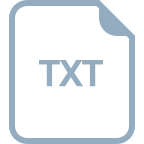
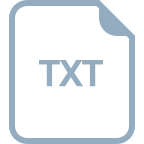
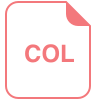
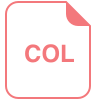
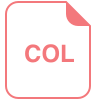
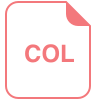










