openssl ECC算法对大文件进行非对称加密和解密
时间: 2023-12-22 22:05:45 浏览: 173
可以使用openssl的ECC算法对大文件进行非对称加密和解密。具体步骤如下:
1. 生成ECC密钥对
使用openssl命令生成ECC密钥对,如下所示:
```
openssl ecparam -name prime256v1 -genkey -noout -out ecc_private.pem
openssl ec -in ecc_private.pem -pubout -out ecc_public.pem
```
这里使用 prime256v1 曲线生成密钥对,ecc_private.pem 是私钥文件,ecc_public.pem 是公钥文件。
2. 加密文件
使用公钥文件对大文件进行加密,如下所示:
```
openssl smime -encrypt -aes256 -in largefile -binary -outform DER -out largefile.enc ecc_public.pem
```
这里使用了AES256对文件进行加密,加密后的文件保存为 largefile.enc。
3. 解密文件
使用私钥文件对加密后的文件进行解密,如下所示:
```
openssl smime -decrypt -in largefile.enc -binary -inform DER -out largefile.dec -inkey ecc_private.pem
```
解密后的文件保存为 largefile.dec。
注意:由于ECC算法加解密速度较慢,对于大文件,加解密时间会比较长。
相关问题
c++ openssl ECC算法对大文件进行非对称加密和解密
可以使用 OpenSSL 库中的 EVP 接口来进行 ECC 算法的非对称加密和解密。以下是一个简单的示例程序,可以对大文件进行加密和解密:
```c++
#include <openssl/evp.h>
#include <openssl/ec.h>
#include <openssl/ecdh.h>
#include <openssl/err.h>
#include <iostream>
#include <fstream>
using namespace std;
int main(int argc, char* argv[])
{
// 读取密钥文件
EC_KEY* ec_key = EC_KEY_new_by_curve_name(NID_secp256k1);
BIO* key_bio = BIO_new_file("private_key.pem", "r");
PEM_read_bio_ECPrivateKey(key_bio, &ec_key, NULL, NULL);
BIO_free(key_bio);
// 初始化加密上下文
EVP_CIPHER_CTX* ctx = EVP_CIPHER_CTX_new();
EVP_CIPHER_CTX_init(ctx);
EVP_EncryptInit_ex(ctx, EVP_ecies(), NULL, NULL, NULL);
EVP_CIPHER_CTX_ctrl(ctx, EVP_CTRL_ECIES_SET_CURVE_NAME, NID_secp256k1, NULL);
EVP_CIPHER_CTX_ctrl(ctx, EVP_CTRL_ECIES_SET_SHARED_INFO_DATA, "SharedInfo", strlen("SharedInfo"), NULL);
// 打开输入文件
ifstream ifs(argv[1], ios::binary | ios::in);
if (!ifs.good()) {
cerr << "Failed to open input file." << endl;
return 1;
}
// 打开输出文件
ofstream ofs(argv[2], ios::binary | ios::out);
if (!ofs.good()) {
cerr << "Failed to open output file." << endl;
return 1;
}
// 循环读取并加密文件数据
unsigned char inbuf[4096], outbuf[4096 + EVP_MAX_CIPHER_BLOCK_SIZE];
int inlen, outlen;
while (!ifs.eof()) {
ifs.read(reinterpret_cast<char*>(inbuf), sizeof(inbuf));
inlen = ifs.gcount();
EVP_EncryptUpdate(ctx, outbuf, &outlen, inbuf, inlen);
ofs.write(reinterpret_cast<char*>(outbuf), outlen);
}
// 结束加密并清理上下文
EVP_EncryptFinal_ex(ctx, outbuf, &outlen);
ofs.write(reinterpret_cast<char*>(outbuf), outlen);
EVP_CIPHER_CTX_free(ctx);
// 关闭文件
ifs.close();
ofs.close();
// 读取密钥文件
ec_key = EC_KEY_new_by_curve_name(NID_secp256k1);
key_bio = BIO_new_file("private_key.pem", "r");
PEM_read_bio_ECPrivateKey(key_bio, &ec_key, NULL, NULL);
BIO_free(key_bio);
// 初始化解密上下文
ctx = EVP_CIPHER_CTX_new();
EVP_CIPHER_CTX_init(ctx);
EVP_DecryptInit_ex(ctx, EVP_ecies(), NULL, NULL, NULL);
EVP_CIPHER_CTX_ctrl(ctx, EVP_CTRL_ECIES_SET_CURVE_NAME, NID_secp256k1, NULL);
EVP_CIPHER_CTX_ctrl(ctx, EVP_CTRL_ECIES_SET_SHARED_INFO_DATA, "SharedInfo", strlen("SharedInfo"), NULL);
// 打开输入文件
ifs.open(argv[2], ios::binary | ios::in);
if (!ifs.good()) {
cerr << "Failed to open input file." << endl;
return 1;
}
// 打开输出文件
ofs.open(argv[3], ios::binary | ios::out);
if (!ofs.good()) {
cerr << "Failed to open output file." << endl;
return 1;
}
// 循环读取并解密文件数据
while (!ifs.eof()) {
ifs.read(reinterpret_cast<char*>(inbuf), sizeof(inbuf));
inlen = ifs.gcount();
EVP_DecryptUpdate(ctx, outbuf, &outlen, inbuf, inlen);
ofs.write(reinterpret_cast<char*>(outbuf), outlen);
}
// 结束解密并清理上下文
EVP_DecryptFinal_ex(ctx, outbuf, &outlen);
ofs.write(reinterpret_cast<char*>(outbuf), outlen);
EVP_CIPHER_CTX_free(ctx);
// 关闭文件
ifs.close();
ofs.close();
return 0;
}
```
在运行程序之前,需要先生成一对 ECC 密钥对,并将私钥写入到文件 private_key.pem 中。可以使用以下命令来生成密钥对:
```
openssl ecparam -name secp256k1 -genkey -noout -out private_key.pem
openssl ec -in private_key.pem -pubout -out public_key.pem
```
在运行程序时,需要指定输入文件、加密后输出文件和解密后输出文件的路径。例如:
```
./ecc_encrypt_decrypt input_file encrypted_file decrypted_file
```
需要注意的是,ECC 算法的加密和解密速度比较慢,对于大文件可能需要等待一些时间。此外,为了保证安全性,需要注意密钥的保护和传输安全等问题。
c++ openssl ECC算法对大文件进行非对称加密和解密,包括生成密钥
好的,这是一个比较复杂的问题,我会尽量详细地回答。
首先,ECC(Elliptic Curve Cryptography,椭圆曲线加密)是一种非对称加密算法,它比传统的 RSA 算法更加高效、安全和灵活。在 OpenSSL 中,可以通过以下步骤对大文件进行 ECC 加密和解密:
1. 生成 ECC 密钥对
可以使用 OpenSSL 中的 EC 函数族来生成 ECC 密钥对。具体步骤如下:
```c++
// 选择一个椭圆曲线
EC_KEY *key = EC_KEY_new_by_curve_name(NID_X9_62_prime256v1);
// 生成密钥对
if (EC_KEY_generate_key(key) != 1) {
// 密钥对生成失败
}
// 获取公钥和私钥
EC_POINT *pub_key = EC_KEY_get0_public_key(key);
BIGNUM *priv_key = EC_KEY_get0_private_key(key);
```
2. 加载公钥和私钥
在加密和解密之前,需要将公钥和私钥加载到内存中。可以使用 OpenSSL 中的 PEM_read_* 函数族从文件中读取密钥,或者使用 d2i_ECPrivateKey 和 i2o_ECPrivateKey 函数直接从内存中读取和写入密钥。
```c++
// 从文件中读取私钥
FILE *fp = fopen("private_key.pem", "r");
EC_KEY *key = PEM_read_ECPrivateKey(fp, NULL, NULL, NULL);
fclose(fp);
// 从内存中读取公钥
unsigned char *pub_key_bytes = ...; // 从文件或网络中读取公钥的二进制表示
EC_KEY *key = EC_KEY_new();
EC_POINT *pub_key = EC_POINT_new(EC_KEY_get0_group(key));
if (EC_POINT_oct2point(EC_KEY_get0_group(key), pub_key, pub_key_bytes, pub_key_len, NULL) != 1) {
// 公钥加载失败
}
if (EC_KEY_set_public_key(key, pub_key) != 1) {
// 公钥设置失败
}
```
3. ECC 加密和解密
在加载完公钥和私钥之后,可以使用 OpenSSL 中的 ECDH 函数族进行 ECC 加密和解密。具体步骤如下:
```c++
// 加密
unsigned char *plaintext = ...; // 待加密的明文
size_t plaintext_len = ...; // 明文长度
unsigned char *ciphertext = (unsigned char *)malloc(EC_GROUP_get_degree(EC_KEY_get0_group(key)) / 8 + plaintext_len);
size_t ciphertext_len = ECDH_compute_key(ciphertext, EC_GROUP_get_degree(EC_KEY_get0_group(key)) / 8, EC_POINT_point2oct(EC_KEY_get0_group(key), EC_KEY_get0_public_key(key), POINT_CONVERSION_UNCOMPRESSED, NULL, 0, NULL), key, NULL);
// 解密
unsigned char *plaintext = (unsigned char *)malloc(ciphertext_len - EC_GROUP_get_degree(EC_KEY_get0_group(key)) / 8);
size_t plaintext_len = ECDH_compute_key(plaintext, ciphertext_len - EC_GROUP_get_degree(EC_KEY_get0_group(key)) / 8, ciphertext, key, NULL);
```
需要注意的是,ECC 加密和解密只能处理长度小于椭圆曲线阶数的数据。对于大文件,可以使用分块加密和解密的方式,即先将文件分成若干个小块,每个小块分别进行加密或解密,最后再将小块拼接起来即可。
希望这个回答能够帮到你。如果还有什么问题,请随时问我。
阅读全文
相关推荐
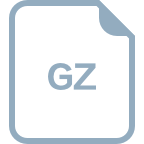
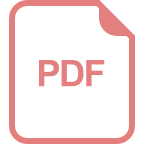



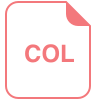
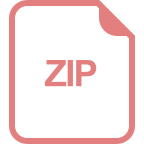
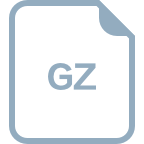
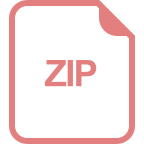
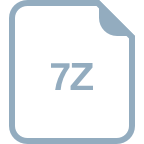
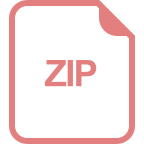
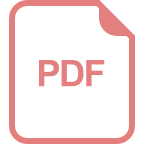
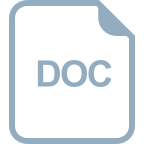
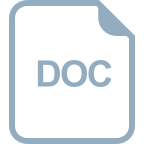
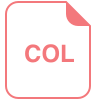

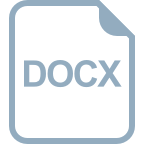