用java 写一个图书管理系统,能够实现输入输出流和ui 界面
时间: 2024-03-26 11:35:22 浏览: 154
好的,我可以给您提供一个简单的图书管理系统的Java代码,包括输入输出流和UI界面。这个系统可以实现添加、删除、查找图书等基本功能。
```java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import javax.swing.*;
public class BookManagementSystem extends JFrame implements ActionListener {
private JTextField tfTitle, tfAuthor, tfPublisher, tfYear, tfSearch;
private JTextArea taDisplay;
private JButton btnAdd, btnDelete, btnSearch, btnClear;
private JPanel panel, panelInput, panelDisplay;
private BufferedReader br;
private BufferedWriter bw;
public BookManagementSystem() {
//设置窗口标题和大小
setTitle("图书管理系统");
setSize(600, 400);
//创建输入输出流
try {
br = new BufferedReader(new FileReader("books.txt"));
bw = new BufferedWriter(new FileWriter("books.txt", true));
} catch (IOException e) {
e.printStackTrace();
}
//创建输入面板
panelInput = new JPanel();
panelInput.setLayout(new GridLayout(5, 2));
panelInput.add(new JLabel("书名:"));
tfTitle = new JTextField();
panelInput.add(tfTitle);
panelInput.add(new JLabel("作者:"));
tfAuthor = new JTextField();
panelInput.add(tfAuthor);
panelInput.add(new JLabel("出版社:"));
tfPublisher = new JTextField();
panelInput.add(tfPublisher);
panelInput.add(new JLabel("出版年份:"));
tfYear = new JTextField();
panelInput.add(tfYear);
btnAdd = new JButton("添加");
btnAdd.addActionListener(this);
panelInput.add(btnAdd);
btnDelete = new JButton("删除");
btnDelete.addActionListener(this);
panelInput.add(btnDelete);
//创建显示面板
panelDisplay = new JPanel();
panelDisplay.setLayout(new BorderLayout());
taDisplay = new JTextArea();
panelDisplay.add(new JScrollPane(taDisplay));
panelDisplay.setBorder(BorderFactory.createTitledBorder("图书列表"));
//创建搜索面板
JPanel panelSearch = new JPanel();
tfSearch = new JTextField(10);
btnSearch = new JButton("搜索");
btnSearch.addActionListener(this);
btnClear = new JButton("清空");
btnClear.addActionListener(this);
panelSearch.add(new JLabel("输入书名:"));
panelSearch.add(tfSearch);
panelSearch.add(btnSearch);
panelSearch.add(btnClear);
//创建主面板
panel = new JPanel();
panel.setLayout(new BorderLayout());
panel.add(panelInput, BorderLayout.NORTH);
panel.add(panelDisplay, BorderLayout.CENTER);
panel.add(panelSearch, BorderLayout.SOUTH);
//将主面板添加到窗口中
add(panel);
//读取文件并显示
try {
String line;
while ((line = br.readLine()) != null) {
taDisplay.append(line + "\n");
}
} catch (IOException e) {
e.printStackTrace();
}
}
//按钮点击事件处理
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnAdd) {
String title = tfTitle.getText();
String author = tfAuthor.getText();
String publisher = tfPublisher.getText();
String year = tfYear.getText();
String book = title + "," + author + "," + publisher + "," + year;
try {
bw.write(book);
bw.newLine();
bw.flush();
} catch (IOException ex) {
ex.printStackTrace();
}
taDisplay.append(book + "\n");
} else if (e.getSource() == btnDelete) {
String title = tfTitle.getText();
String line;
try {
File file = new File("books.txt");
BufferedReader br = new BufferedReader(new FileReader(file));
StringBuffer sb = new StringBuffer("");
while ((line = br.readLine()) != null) {
if (line.indexOf(title) == -1) {
sb.append(line);
sb.append("\r\n");
}
}
br.close();
FileWriter fw = new FileWriter(file);
fw.write(sb.toString());
fw.close();
JOptionPane.showMessageDialog(null, "删除成功!");
} catch (Exception ex) {
ex.printStackTrace();
}
} else if (e.getSource() == btnSearch) {
String title = tfSearch.getText();
taDisplay.setText("");
try {
String line;
while ((line = br.readLine()) != null) {
if (line.indexOf(title) != -1) {
taDisplay.append(line + "\n");
}
}
} catch (IOException ex) {
ex.printStackTrace();
}
} else if (e.getSource() == btnClear) {
tfSearch.setText("");
taDisplay.setText("");
try {
String line;
br = new BufferedReader(new FileReader("books.txt"));
while ((line = br.readLine()) != null) {
taDisplay.append(line + "\n");
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
public static void main(String[] args) {
BookManagementSystem frame = new BookManagementSystem();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
此代码实现了基本的图书管理系统的功能,包括添加、删除、查找和清空等操作。它使用了输入输出流读写文件,并使用了Java Swing库创建了一个简单的UI界面。请注意,此代码仅作为参考,实际项目中可能需要更多的功能和更复杂的实现方式。
阅读全文
相关推荐
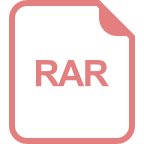


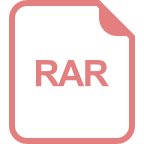
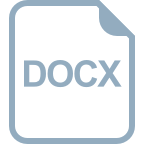
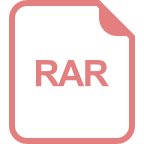
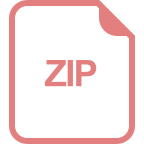
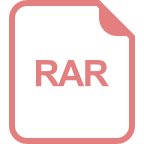
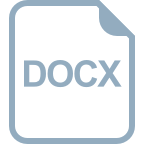
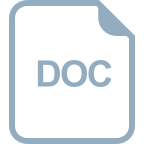
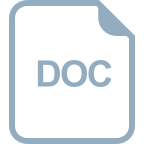
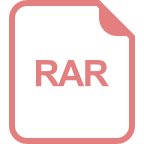
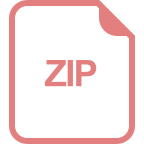
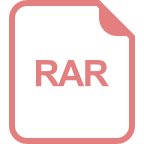
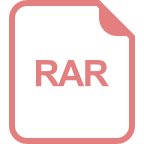
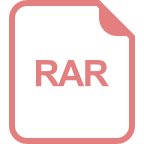
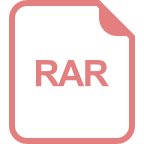