用c语言从键盘读取用户输入直到遇到#字符,编写程序统计读取的空格数目、读取的换行符数目以及读取的所有其他字符数目。(要求用getchar()输入字符) 程序运行结果示例1: Please input a string end by #: abc def↙ jklm op↙ zkm #↙ space: 3,newline: 2,others: 15 程序运行结果示例2: Please input a string end by #: hello friend!#↙ space: 1,newline: 0
时间: 2023-12-03 18:45:59 浏览: 33
下面是实现上述功能的 C 语言程序:
```c
#include <stdio.h>
int main() {
char c;
int space_count = 0, newline_count = 0, other_count = 0;
printf("Please input a string end by #: ");
while ((c = getchar()) != '#') {
if (c == ' ') {
space_count++;
} else if (c == '\n') {
newline_count++;
} else {
other_count++;
}
}
printf("space: %d, newline: %d, others: %d\n", space_count, newline_count, other_count);
return 0;
}
```
程序的主要思路是利用 `getchar()` 函数从标准输入读取字符,遇到 `#` 字符就结束循环,并统计空格、换行和其他字符的个数。最后输出这些统计结果。
需要注意的是,由于输入的字符可能包括空格和换行符,因此在读取字符时应该使用 `char` 类型,而不是 `int` 类型。此外,程序还需要声明并初始化三个计数器变量,用于统计不同类型的字符个数。
相关问题
用C语言编写一个程序,从键盘读取用户输入直到遇到#字符,编写程序统计读取的空格数目、读取的换行符数目以及读取的所有其他字符数目。(要求用getchar()输入字符) 程序运行结果示例1: Please input a string end by #: abc def↙ jklm op↙ zkm #↙ space: 3,newline: 2,others: 15 程序运行结果示例2: Please input a string end by #: hello friend!#↙ space: 1,new
line: 0,others: 13
```c
#include <stdio.h>
int main() {
char c;
int space = 0, newline = 0, others = 0;
printf("Please input a string end by #: ");
while ((c = getchar()) != '#') {
if (c == ' ') {
space++;
} else if (c == '\n') {
newline++;
} else {
others++;
}
}
printf("space: %d, newline: %d, others: %d\n", space, newline, others);
return 0;
}
```
C语言:编程从键盘输入若干字符,直到输入字符#为止,统计其中的大写字母字符、小写字母字符、数字字符、空白符(空格、制表符和换行符统称空白符) 以及其他字符的个数并输出统计结果。
这个问题可以沿用上一个问题中的思路,只需要增加一个计数器变量用于统计空白符的数量,以及一个计数器变量用于统计其他字符的数量。具体的实现如下:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
int upper_count = 0; // 大写字母计数器
int lower_count = 0; // 小写字母计数器
int digit_count = 0; // 数字字符计数器
int space_count = 0; // 空白符计数器
int other_count = 0; // 其他字符计数器
char ch; // 输入的字符
printf("请输入若干字符,以#结束:\n");
while ((ch = getchar()) != '#') {
if (isupper(ch)) {
upper_count++;
} else if (islower(ch)) {
lower_count++;
} else if (isdigit(ch)) {
digit_count++;
} else if (isspace(ch)) {
space_count++;
} else {
other_count++;
}
}
printf("大写字母数量:%d\n", upper_count);
printf("小写字母数量:%d\n", lower_count);
printf("数字字符数量:%d\n", digit_count);
printf("空白符数量:%d\n", space_count);
printf("其他字符数量:%d\n", other_count);
return 0;
}
```
在这个程序中,我们增加了两个计数器变量,分别用于统计空白符和其他字符的数量。如果读取到的字符是空白符,则将空白符计数器加 1;如果读取到的字符不是大写字母、小写字母、数字字符或空白符,则将其他字符计数器加 1。最后输出所有计数器变量的值即可。
相关推荐
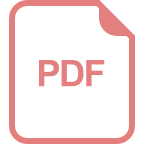
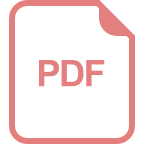
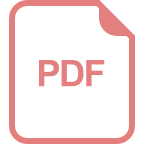
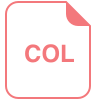











