为一维数组输入10个整数;将其中最小的数与第一个数对换,将最大的数与最后一个数对换;输出数组元素。。
时间: 2023-05-31 15:01:54 浏览: 177
### 回答1:
好的,以下是用中文回复:
1. 首先,我们需要输入10个整数,可以使用循环语句来实现:
```
int arr[10];
for(int i=; i<10; i++){
cin >> arr[i];
}
```
2. 接下来,我们需要找到最小值和最大值的下标,可以使用循环遍历整个数组,找到最小值和最大值的下标:
```
int minIndex = , maxIndex = ;
for(int i=1; i<10; i++){
if(arr[i] < arr[minIndex]){
minIndex = i;
}
if(arr[i] > arr[maxIndex]){
maxIndex = i;
}
}
```
3. 然后,我们将最小值和第一个数对换,将最大值和最后一个数对换:
```
int temp = arr[];
arr[] = arr[minIndex];
arr[minIndex] = temp;
temp = arr[9];
arr[9] = arr[maxIndex];
arr[maxIndex] = temp;
```
4. 最后,我们输出数组元素:
```
for(int i=; i<10; i++){
cout << arr[i] << " ";
}
```
完整代码如下:
```
int arr[10];
for(int i=; i<10; i++){
cin >> arr[i];
}
int minIndex = , maxIndex = ;
for(int i=1; i<10; i++){
if(arr[i] < arr[minIndex]){
minIndex = i;
}
if(arr[i] > arr[maxIndex]){
maxIndex = i;
}
}
int temp = arr[];
arr[] = arr[minIndex];
arr[minIndex] = temp;
temp = arr[9];
arr[9] = arr[maxIndex];
arr[maxIndex] = temp;
for(int i=; i<10; i++){
cout << arr[i] << " ";
}
``` ### 回答2:
这道题的思路是比较简单的,我们可以利用循环遍历数组,先找到最小的数和最大的数。然后再分别与第一个数和最后一个数进行交换。
下面是具体的步骤:
1. 首先,我们需要定义一个长度为10的一维数组,用来存储这10个整数:
```
int[] arr = new int[10];
```
2. 然后,我们需要通过循环读取用户输入的10个整数,并保存到数组中:
```
for (int i = 0; i < 10; i++) {
System.out.println("请输入第" + (i+1) + "个整数:");
arr[i] = scanner.nextInt();
}
```
3. 接下来,我们需要找到最小的数和最大的数:
```
int minIndex = 0; // 最小值的下标
int maxIndex = 0; // 最大值的下标
for (int i = 0; i < 10; i++) {
if (arr[i] < arr[minIndex]) { // 找到最小值
minIndex = i;
}
if (arr[i] > arr[maxIndex]) { // 找到最大值
maxIndex = i;
}
}
```
4. 然后,我们需要交换最小值与第一个数,最大值与最后一个数:
```
int temp = arr[0];
arr[0] = arr[minIndex];
arr[minIndex] = temp;
temp = arr[9];
arr[9] = arr[maxIndex];
arr[maxIndex] = temp;
```
5. 最后,我们需要输出数组元素:
```
for (int i = 0; i < 10; i++) {
System.out.print(arr[i] + " ");
}
```
完整代码如下:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int[] arr = new int[10];
for (int i = 0; i < 10; i++) {
System.out.println("请输入第" + (i+1) + "个整数:");
arr[i] = scanner.nextInt();
}
int minIndex = 0; // 最小值的下标
int maxIndex = 0; // 最大值的下标
for (int i = 0; i < 10; i++) {
if (arr[i] < arr[minIndex]) { // 找到最小值
minIndex = i;
}
if (arr[i] > arr[maxIndex]) { // 找到最大值
maxIndex = i;
}
}
int temp = arr[0];
arr[0] = arr[minIndex];
arr[minIndex] = temp;
temp = arr[9];
arr[9] = arr[maxIndex];
arr[maxIndex] = temp;
for (int i = 0; i < 10; i++) {
System.out.print(arr[i] + " ");
}
scanner.close();
}
}
```
### 回答3:
题目要求我们编写一个程序,先要求输入10个整数,然后将其中最小的数与第一个数对换,将最大的数与最后一个数对换,最后输出数组元素。
首先,我们需要用一个循环语句依次输入10个整数,存储在一个数组中。例如:
```python
arr = []
for i in range(10):
num = int(input("请输入第%d个整数:" % (i+1)))
arr.append(num)
```
接下来,我们需要找到最小值和最大值的下标,然后进行对换。可以先定义两个变量min_index和max_index来存储最小值和最大值的下标,然后用一个循环语句遍历整个数组,找到最小值和最大值的下标。例如:
```python
min_index = 0
max_index = 0
for i in range(1, 10):
if arr[i] < arr[min_index]:
min_index = i
if arr[i] > arr[max_index]:
max_index = i
# 将最小的数与第一个数对换
arr[0], arr[min_index] = arr[min_index], arr[0]
# 将最大的数与最后一个数对换
arr[-1], arr[max_index] = arr[max_index], arr[-1]
```
最后,我们通过循环语句输出对换后的数组元素即可,例如:
```python
for i in range(10):
print(arr[i])
```
完整的程序如下:
```python
arr = []
for i in range(10):
num = int(input("请输入第%d个整数:" % (i+1)))
arr.append(num)
min_index = 0
max_index = 0
for i in range(1, 10):
if arr[i] < arr[min_index]:
min_index = i
if arr[i] > arr[max_index]:
max_index = i
# 将最小的数与第一个数对换
arr[0], arr[min_index] = arr[min_index], arr[0]
# 将最大的数与最后一个数对换
arr[-1], arr[max_index] = arr[max_index], arr[-1]
for i in range(10):
print(arr[i])
```
阅读全文
相关推荐
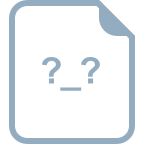
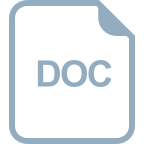
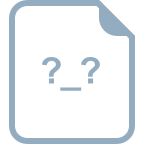
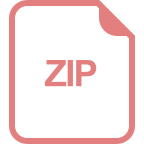
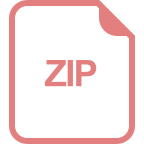
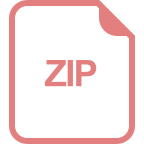
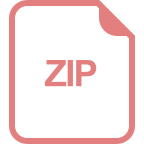
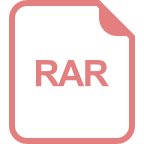
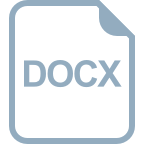
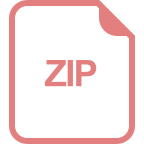
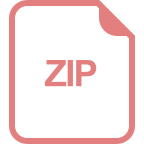