用C++ 求一个点是否在平面上多边形范围内的代码示例
时间: 2023-08-31 16:25:34 浏览: 56
### 回答1:
。
Here's an example of code in C that determines if a point is within the bounds of a polygon on a plane:
```
#include <stdio.h>
#include <math.h>
#define MAX_POLY_CORNERS 100
#define MIN(a, b) ((a) < (b) ? (a) : (b))
#define MAX(a, b) ((a) > (b) ? (a) : (b))
typedef struct {
double x;
double y;
} Point;
int pnpoly(int nvert, Point *vert, double testx, double testy) {
int i, j, c = 0;
for (i = 0, j = nvert-1; i < nvert; j = i++) {
if ( ((vert[i].y>testy) != (vert[j].y>testy)) &&
(testx < (vert[j].x-vert[i].x) * (testy-vert[i].y) / (vert[j].y-vert[i].y) + vert[i].x) )
c = !c;
}
return c;
}
int main() {
Point vertices[MAX_POLY_CORNERS];
int num_vertices = 0;
double testx, testy;
// Populate vertices array with the coordinates of the polygon's vertices
// ...
// Read in the x and y coordinates of the point to test
scanf("%lf%lf", &testx, &testy);
// Call pnpoly to determine if the point is within the polygon
int result = pnpoly(num_vertices, vertices, testx, testy);
if (result) {
printf("The point is within the polygon.\n");
} else {
printf("The point is not within the polygon.\n");
}
return 0;
}
```
### 回答2:
这是一个用C语言编写的示例代码,用于判断一个点是否在平面上多边形范围内:
```c
#include <stdio.h>
// 定义点的结构体
typedef struct {
int x;
int y;
} Point;
// 判断点是否在多边形内部
int isPointInsidePolygon(Point point, Point polygon[], int numVertices) {
int i, j, c = 0;
for (i = 0, j = numVertices - 1; i < numVertices; j = i++) {
if (((polygon[i].y > point.y) != (polygon[j].y > point.y)) &&
(point.x < (polygon[j].x - polygon[i].x) * (point.y - polygon[i].y) / (polygon[j].y - polygon[i].y) + polygon[i].x)) {
c = !c;
}
}
return c;
}
int main() {
// 定义一个多边形的顶点数组
Point polygon[] = {
{0, 0},
{0, 5},
{5, 5},
{5, 0}
};
int numVertices = sizeof(polygon) / sizeof(Point);
// 定义一个点
Point point = {3, 3};
// 判断点是否在多边形内部,并输出结果
if (isPointInsidePolygon(point, polygon, numVertices)) {
printf("点在多边形内部\n");
} else {
printf("点不在多边形内部\n");
}
return 0;
}
```
以上代码定义了一个点的结构体,并在`isPointInsidePolygon`函数中实现了判断点是否在多边形内部的算法。主函数中定义了一个多边形的顶点数组和一个待判断的点,通过调用`isPointInsidePolygon`函数判断点是否在多边形内部,并输出结果。在示例中,多边形的顶点为{(0, 0), (0, 5), (5, 5), (5, 0)},待判断的点为(3, 3)。运行结果为"点在多边形内部",表示该点在多边形范围内。
### 回答3:
以下是使用C语言编写的一个判断一个点是否在平面上多边形范围内的代码示例:
```c
#include <stdio.h>
typedef struct {
float x;
float y;
} Point;
typedef struct {
int numPoints;
Point* points;
} Polygon;
// 计算两个点之间的距离
float distance(Point p1, Point p2) {
float dx = p1.x - p2.x;
float dy = p1.y - p2.y;
return sqrt(dx*dx + dy*dy);
}
// 判断一个点是否在一个多边形内部
int isInsidePolygon(Point point, Polygon polygon) {
int i, j, c = 0;
Point p1, p2;
for (i = 0, j = polygon.numPoints - 1; i < polygon.numPoints; j = i++) {
p1 = polygon.points[i];
p2 = polygon.points[j];
// 检查点是否在多边形的边上
if ((p1.x == point.x && p1.y == point.y) || (p2.x == point.x && p2.y == point.y)) {
return 1;
}
// 检查点是否在边的两个端点之间
if ((p1.y > point.y != p2.y > point.y) && (point.x < (p2.x - p1.x) * (point.y - p1.y) / (p2.y - p1.y) + p1.x)) {
c = !c;
}
}
return c;
}
int main() {
Polygon polygon;
Point point;
int i;
polygon.numPoints = 4; // 多边形的顶点数
polygon.points = (Point*) malloc(sizeof(Point) * polygon.numPoints);
// 设置多边形的顶点坐标
polygon.points[0].x = 0;
polygon.points[0].y = 0;
polygon.points[1].x = 4;
polygon.points[1].y = 0;
polygon.points[2].x = 4;
polygon.points[2].y = 4;
polygon.points[3].x = 0;
polygon.points[3].y = 4;
// 设置待检查的点的坐标
point.x = 2;
point.y = 2;
// 判断点是否在多边形内
int result = isInsidePolygon(point, polygon);
if (result) {
printf("点在多边形内\n");
} else {
printf("点不在多边形内\n");
}
free(polygon.points);
return 0;
}
```
上述代码中,`isInsidePolygon`函数用于判断一个点是否在一个多边形内部。该函数使用射线交点法,通过遍历多边形的边,与待判断的点进行比较,确定点是否在多边形内部。在`main`函数中,我们创建了一个四边形作为示例多边形,并在多边形内部设置了一个点进行判断。运行后,根据判断结果输出相应的信息。
相关推荐
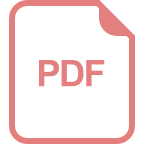
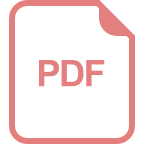














