1、以顺序表为例,设计一个主程序完成如下功能:e 1)初始化顺序表L 2)依饮插入a,b.c,d.e元素 3)输出L的长度一 4)判断L是否为空。 5)输出L的第3个元素。 6)输出元素b的位置
时间: 2024-11-30 17:14:17 浏览: 16
在C++中,你可以使用结构体(struct)表示顺序表,数组存储元素,然后编写一个函数来完成这些操作。以下是简单的示例:
```cpp
#include <iostream>
using namespace std;
// 定义顺序表元素结构
struct Element {
char data;
int position; // 存储元素位置
};
// 顺序表类型定义
typedef struct Element* SequenceList;
// 初始化顺序表
void initialize(SequenceList& L, int size) {
L = new Element[size];
for (int i = 0; i < size; ++i) {
L[i].data = '\0';
L[i].position = -1; // 空位标记
}
}
// 插入元素到顺序表
void insert(SequenceList& L, char element) {
for (int i = 0; L[i].position != -1; ++i) {
if (L[i].data == '\0') {
L[i].data = element;
L[i].position = i + 1;
break;
}
}
}
// 其他函数以此类推
int length(SequenceList L) { return (L[0].position == -1 ? 0 : L[0].position); }
bool isEmpty(SequenceList L) { return length(L) == 0; }
char getThirdElement(SequenceList L) {
if (length(L) >= 3) {
return L[2].data;
} else {
cout << "Error: List is too short to access the third element." << endl;
return '\0';
}
}
int getPosition(char element, SequenceList L) {
for (int i = 0; L[i].data != '\0'; ++i) {
if (L[i].data == element) {
return L[i].position;
}
}
cout << "Element not found in the list." << endl;
return -1;
}
int main() {
const int capacity = 5;
SequenceList L = new Element[capacity];
// 初始化和插入元素
initialize(L, capacity);
insert(L, 'a');
insert(L, 'b');
insert(L, 'c');
insert(L, 'd');
insert(L, 'e');
// 输出结果
cout << "Length of the list: " << length(L) << endl;
cout << "Is the list empty? " << (isEmpty(L) ? "Yes" : "No") << endl;
cout << "The third element: " << getThirdElement(L) << endl;
cout << "Position of element 'b': " << getPosition('b', L) << endl;
delete[] L;
return 0;
}
```
请注意,这个例子假设你有足够的内存空间来创建顺序表,并且没有对用户输入的有效性进行检查。实际应用中,你需要添加适当的错误处理和边界条件检查。
阅读全文
相关推荐
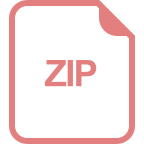
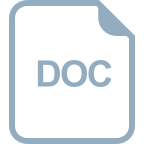
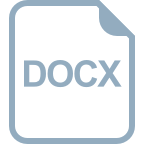















