用C语言以顺序表为例,设计一个主程序完成如下功能: 1)初始化顺序表L 2)依次插入a,b,c,d,e元素 3)输出L的长度 4)判断L是否为空 5)输出L的第3个元素 6)输出元素b的位置
时间: 2024-09-13 09:14:52 浏览: 58
在C语言中,我们可以使用结构体和数组来创建一个简单的顺序表。首先,我们定义一个结构体`Node`表示列表节点,并包含一个整型数据和指向下一个节点的指针。接下来,我会给出一个示例如何实现上述功能。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化顺序表函数
void initList(Node** L) {
*L = (Node*)malloc(sizeof(Node));
if (*L == NULL) {
printf("内存分配失败\n");
exit(1);
}
(*L)->next = NULL;
}
// 插入元素到顺序表函数
void insertElement(Node** L, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("内存分配失败\n");
exit(1);
}
newNode->data = value;
newNode->next = *L;
*L = newNode;
}
// 计算并输出列表长度
int listLength(Node* L) {
int length = 0;
Node* current = L;
while (current != NULL) {
length++;
current = current->next;
}
return length;
}
// 判断列表是否为空
int isEmpty(Node* L) {
return L == NULL;
}
// 输出第n个元素(从0开始计数)
int getNthElement(Node* L, int n) {
for (int i = 0; i < n && L != NULL; i++) {
L = L->next;
}
return L ? L->data : -1; // 如果找到返回值,否则返回-1
}
// 输出元素b的位置
int getPosition(Node* L, int b) {
int position = 0;
Node* current = L;
while (current != NULL && current->data != b) {
position++;
current = current->next;
}
return position;
}
int main() {
Node* L = NULL;
// 1)初始化顺序表L
initList(&L);
// 2)依次插入a, b, c, d, e元素
insertElement(&L, 'a');
insertElement(&L, 'b');
insertElement(&L, 'c');
insertElement(&L, 'd');
insertElement(&L, 'e');
// 3)输出L的长度
printf("列表长度: %d\n", listLength(L));
// 4)判断L是否为空
if (isEmpty(L)) {
printf("列表为空\n");
} else {
printf("列表不为空\n");
}
// 5)输出L的第3个元素
int thirdElement = getNthElement(L, 2); // 注意索引从0开始
if (thirdElement != -1)
printf("第3个元素: %c\n", thirdElement);
else
printf("无法获取第3个元素\n");
// 6)输出元素b的位置
int bPosition = getPosition(L, 'b');
printf("元素'b'的位置: %d\n", bPosition);
return 0;
}
```
阅读全文
相关推荐
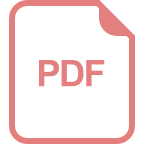
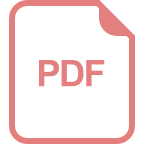
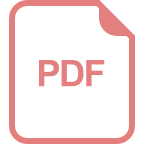

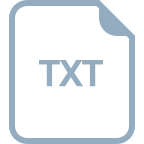
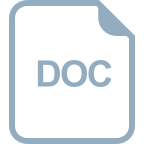
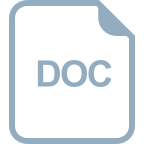
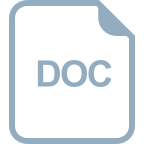
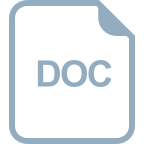
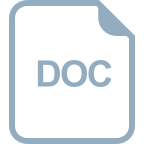
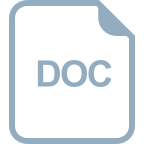
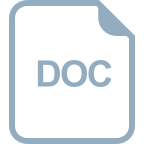






