c语言 ,设计实现一个顺序表,具有初始化、插入、删除、显示及取值等功能。
时间: 2024-09-26 19:12:51 浏览: 55
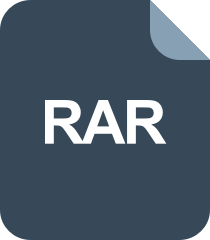
上海交大 C语言程序设计课件.rar
在C语言中,我们可以使用结构体(struct)和指针来设计一个简单的顺序表,这里我会给出一个基本的顺序表(链式存储)实现示例,它包含初始化、插入、删除、显示元素和取值的功能。
首先,我们定义一个节点结构:
```c
typedef struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
} Node;
```
然后,创建顺序表的结构:
```c
typedef struct List {
Node* head; // 链表头指针
int size; // 列表长度
} List;
```
接下来,我们实现初始化函数`init_list`:
```c
void init_list(List* list) {
list->head = NULL;
list->size = 0;
}
```
插入操作`insert`:
```c
void insert(List* list, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = list->head;
list->head = newNode;
list->size++;
}
```
删除操作`delete`(这里假设我们要删除的是第一个元素):
```c
void delete(List* list) {
if (list->head != NULL) {
Node* temp = list->head;
list->head = list->head->next;
free(temp);
list->size--;
}
}
```
显示元素的函数`display`:
```c
void display(List* list) {
Node* current = list->head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
获取指定位置的值`get_value`(假设从0开始计数):
```c
int get_value(List* list, int index) {
if (index < 0 || index >= list->size) {
return -1; // 或者抛出错误,表示索引越界
}
Node* current = list->head;
for (int i = 0; i < index; i++) {
current = current->next;
}
return current->data;
}
```
以上就是基本的顺序表实现。使用时,需要先调用`init_list`初始化列表,然后按照需要调用其他功能函数。
阅读全文
相关推荐
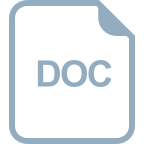
















