用c-free写一个顺序表代码要求顺序表中包含初始化取值查找插入删除
时间: 2024-11-17 07:29:20 浏览: 18
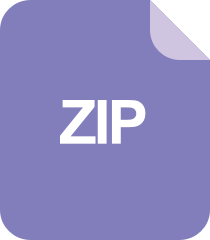
C-Programming-Project
在CFree(一种用于教学目的的C语言环境)中,我们可以创建一个简单的顺序表结构来实现初始化、取值、查找、插入和删除操作。首先,我们定义一个链式节点结构和一个顺序表结构:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链式节点
typedef struct Node {
int data;
struct Node* next;
} Node;
// 定义顺序表
typedef struct List {
Node* head;
int size;
} List;
// 初始化列表
List* create_list() {
List* list = (List*)malloc(sizeof(List));
list->head = NULL;
list->size = 0;
return list;
}
// 在列表末尾插入元素
void insert(List* list, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
} else {
Node* current = list->head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
list->size++;
}
// 查找元素
int find(List* list, int value) {
Node* current = list->head;
for (int i = 0; i < list->size && current != NULL; i++, current = current->next) {
if (current->data == value) {
return i;
}
}
return -1; // 如果未找到,返回-1
}
// 删除元素
void remove(List* list, int index) {
if (index >= 0 && index < list->size) {
if (list->head == NULL) {
return;
} else if (index == 0) {
Node* temp = list->head;
list->head = list->head->next;
free(temp);
} else {
Node* current = list->head;
for (int i = 0; i < index - 1; i++) {
current = current->next;
}
Node* toRemove = current->next;
current->next = toRemove->next;
free(toRemove);
}
list->size--;
} else {
printf("Invalid index.\n");
}
}
// 打印列表
void print_list(List* list) {
Node* current = list->head;
for (int i = 0; i < list->size; i++, current = current->next) {
printf("%d ", current->data);
}
printf("\n");
}
int main() {
List* myList = create_list();
insert(myList, 5);
insert(myList, 10);
insert(myList, 15);
print_list(myList);
int position = find(myList, 10);
if (position != -1) {
printf("Found element at position %d\n", position + 1);
remove(myList, position); // 删除位置10的元素
} else {
printf("Element not found.\n");
}
print_list(myList);
return 0;
}
```
在这个代码示例中,我们实现了顺序表的基本操作。运行`main`函数可以看到初始化、插入、查找和删除的操作效果。请注意,这只是一个基础版本,实际项目中可能需要考虑更多的边界情况和错误处理。
阅读全文
相关推荐
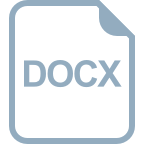
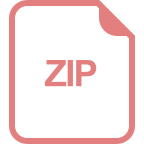











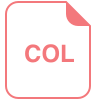

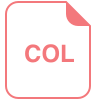
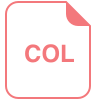
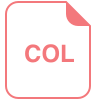