写一个满足线性表顺序存储结构下基本操作的实现(初始化、赋值、取值、查找、插入、删除、两个非递减有序顺序表的归并等)的c代码
时间: 2024-09-20 18:01:31 浏览: 22
下面是一个简单的线性表顺序存储结构的 C 语言实现,包括了初始化、赋值、取值、查找、插入、删除以及两个有序链表的归并操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化空链表
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node));
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->next = NULL;
return head;
}
// 插入元素到链表末尾
void insert(int value, Node** head) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
// 查找元素
int search(int target, Node* head) {
Node* current = head;
while (current != NULL) {
if (current->data == target)
return 1; // 找到了
current = current->next;
}
return 0; // 没找到
}
// 删除元素
void deleteElement(int target, Node** head) {
if (*head == NULL)
return;
if ((*head)->data == target) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* prev = *head;
Node* current = (*head)->next;
while (current != NULL && current->data != target) {
prev = current;
current = current->next;
}
if (current != NULL) {
prev->next = current->next;
free(current);
}
}
// 合并两个升序链表
Node* mergeSortedLists(Node* list1, Node* list2) {
if (list1 == NULL)
return list2;
else if (list2 == NULL)
return list1;
if (list1->data <= list2->data) {
list1->next = mergeSortedLists(list1->next, list2);
return list1;
} else {
list2->next = mergeSortedLists(list1, list2->next);
return list2;
}
}
// 打印链表
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
int main() {
Node* list1 = createList();
Node* list2 = createList();
// 插入、查找、删除操作示例...
insert(5, &list1);
insert(3, &list2);
printList(list1); // 输出: 5 ->
printf("Searching for 5: %d\n", search(5, list1)); // 输出: 1
deleteElement(3, &list2);
printList(list2); // 输出: 5 ->
list1 = mergeSortedLists(list1, list2);
printList(list1); // 输出: 3 5 ->
return 0;
}
```
这个代码展示了如何创建链表、插入元素、搜索、删除元素以及合并两个有序链表的基本操作。注意,这只是一个简化版的实现,实际应用中还需要处理更多边界情况和错误检查。
相关推荐
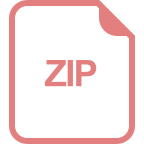
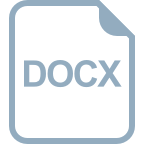















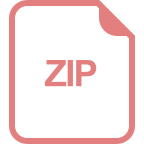
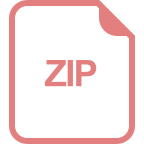