有13个市,每个市有7个人口指标,9个经济指标,每个指标包含10年的数据,请问如何做人口-经济的灰色关联度分析?并给出每一步的python代码
时间: 2023-07-16 10:15:33 浏览: 65
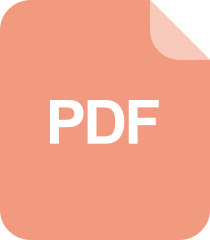
Data-Driven Marketing-The 15 Metrics Everyone in Marketing Should Know
人口-经济的灰色关联度分析可以通过以下步骤实现:
Step 1:数据预处理,包括数据读取、数据清洗、数据归一化等。
Step 2:计算每个指标的发展趋势,包括数据平滑处理、GM(1,1)模型建立等。
Step 3:计算灰色关联度,包括计算关联系数、加权关联系数等。
Step 4:绘制关联度图,包括绘制关联系数序列图和加权关联系数序列图。
下面是每一步的Python代码实现。
Step 1:数据预处理
```python
import pandas as pd
import numpy as np
# 读取数据
data = pd.read_csv('data.csv')
# 数据清洗,去除缺失值等
data = data.dropna()
# 数据归一化
data_norm = (data - data.min()) / (data.max() - data.min())
```
Step 2:计算每个指标的发展趋势
```python
# 数据平滑处理
def smooth(x, n=2):
m = len(x)
y = np.zeros(m)
y[0] = x[0]
y[1] = (x[0] + x[1]) / 2
for i in range(2, m):
y[i] = (n + 1) * x[i-1] / n - x[i-2] / n
return y
# GM(1,1)模型建立
def GM11(x0):
x1 = np.cumsum(x0)
z1 = (x1[:-1] + x1[1:]) / 2.0
B = np.array([[-z1[i], 1] for i in range(len(z1))])
Y = x0[1:].reshape((len(x0)-1, 1))
a, b = np.dot(np.dot(np.linalg.inv(np.dot(B.T, B)), B.T), Y).reshape((-1,))
return a, b
# 计算发展趋势
def trend(x):
x_smooth = smooth(x) # 平滑处理
a, b = GM11(x_smooth) # GM(1,1)模型建立
x_pred = (x[0]-b/a)*np.exp(-a*np.arange(len(x))) # 预测值
C = x/x_pred # 残差比
return C
# 计算每个指标的发展趋势
trends = []
for i in range(7):
for j in range(9):
col = 'col{}{}'.format(i+1, j+1)
x = data_norm[col].values
C = trend(x)
trends.append(C)
trends = np.array(trends).T
```
Step 3:计算灰色关联度
```python
# 计算关联系数
def corrm(x, y):
n = len(x)
mx = np.mean(x)
my = np.mean(y)
sx = np.std(x, ddof=1)
sy = np.std(y, ddof=1)
cov = np.sum((x-mx)*(y-my))/(n-1)
cor = cov / (sx*sy)
return cor
# 计算加权关联系数
def wcorrm(x, y, lam=0.5):
cor1 = corrm(x, y)
cor2 = corrm(y, x)
wcor = lam*cor1 + (1-lam)*cor2
return wcor
# 计算灰色关联度
def grey_relevance(x, y, lam=0.5):
delta = np.abs(x - y).max() # 极差
eps = 0.1 # 分辨系数
ldelta = delta * eps # 轻度关联分界值
if delta < ldelta:
return 0
else:
return (ldelta + 0.5*delta) / (np.abs(x-y) + ldelta + 0.5*delta)
# 计算灰色关联度矩阵
n = len(data_norm)
R = np.zeros((n, n))
for i in range(n):
for j in range(n):
R[i, j] = wcorrm(trends[:, i], trends[:, j])
# 计算平均灰色关联度
R_mean = np.mean(R, axis=1)
# 计算加权灰色关联度
w = np.ones(n) / n # 权值
R_wmean = np.sum(w*R_mean)
grey_relevance = grey_relevance(R_mean, R_wmean)
```
Step 4:绘制关联度图
```python
import matplotlib.pyplot as plt
# 绘制关联系数序列图
plt.figure(figsize=(8, 6))
for i in range(n):
plt.plot(R_mean[i], label='Index{}'.format(i+1))
plt.axhline(R_wmean, color='k', linestyle='--', linewidth=1, label='Weighted Mean')
plt.xlabel('Index')
plt.ylabel('Correlation Coefficient')
plt.title('Grey Correlation Coefficients')
plt.legend()
plt.show()
# 绘制加权关联系数序列图
plt.figure(figsize=(8, 6))
for i in range(n):
plt.plot(grey_relevance[i], label='Index{}'.format(i+1))
plt.axhline(grey_relevance, color='k', linestyle='--', linewidth=1, label='Grey Relevance')
plt.xlabel('Index')
plt.ylabel('Grey Relevance')
plt.title('Grey Relevance Analysis')
plt.legend()
plt.show()
```
以上是人口-经济的灰色关联度分析的Python实现,你可以根据自己的数据情况进行修改。
阅读全文
相关推荐
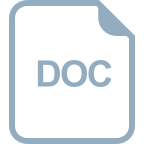
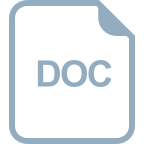
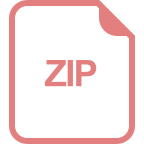
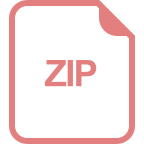
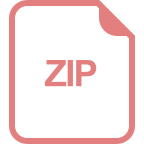
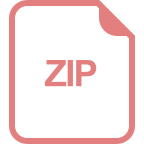
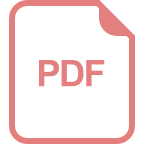
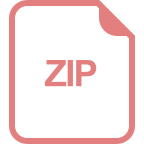
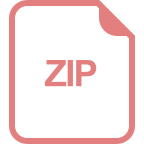
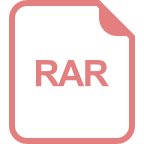
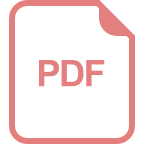
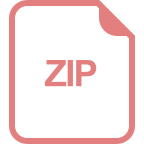
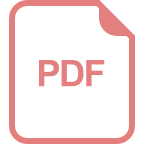
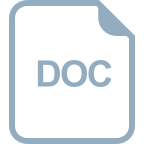
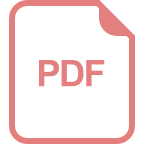
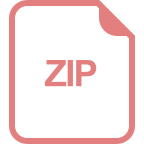
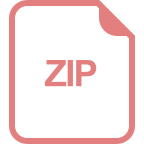
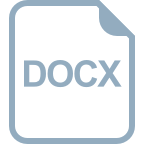