用c语言写一个叫号系统
时间: 2023-10-10 15:08:44 浏览: 88
好的,下面是用 C 语言实现一个简单的叫号系统的代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
// 定义队列结构体
typedef struct {
int items[MAX_SIZE];
int front;
int rear;
} Queue;
// 初始化队列
void initQueue(Queue* queue) {
queue->front = -1;
queue->rear = -1;
}
// 判断队列是否为空
int isQueueEmpty(Queue* queue) {
return queue->front == -1 && queue->rear == -1;
}
// 判断队列是否已满
int isQueueFull(Queue* queue) {
return queue->rear == MAX_SIZE - 1;
}
// 入队操作
void enqueue(Queue* queue, int value) {
if (isQueueFull(queue)) {
printf("队列已满,无法入队!\n");
return;
}
if (isQueueEmpty(queue)) {
queue->front = 0;
queue->rear = 0;
} else {
queue->rear++;
}
queue->items[queue->rear] = value;
}
// 出队操作
int dequeue(Queue* queue) {
if (isQueueEmpty(queue)) {
printf("队列为空,无法出队!\n");
return -1;
}
int value = queue->items[queue->front];
if (queue->front == queue->rear) {
queue->front = -1;
queue->rear = -1;
} else {
queue->front++;
}
return value;
}
int main() {
Queue queue;
initQueue(&queue);
int lastNumber = 0;
char choice;
do {
printf("请输入选项:\n");
printf("1. 取号\n");
printf("2. 呼叫\n");
printf("3. 退出\n");
scanf(" %c", &choice);
switch (choice) {
case '1':
lastNumber++;
printf("请记住您的号码:%d\n", lastNumber);
enqueue(&queue, lastNumber);
break;
case '2':
if (isQueueEmpty(&queue)) {
printf("队列为空,无法呼叫!\n");
} else {
int number = dequeue(&queue);
printf("请 %d 号到窗口办理业务!\n", number);
}
break;
case '3':
printf("谢谢使用叫号系统!\n");
break;
default:
printf("输入有误,请重新输入!\n");
break;
}
} while (choice != '3');
return 0;
}
```
该叫号系统使用队列实现,最大长度为 100。程序运行时,用户可以选择取号、呼叫或退出。取号操作会自动为用户分配一个号码,并将其加入队列中;呼叫操作会从队列中取出一个号码进行呼叫。在 main() 函数中通过一个 do-while 循环实现了用户交互的过程。
相关推荐
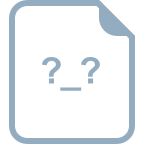













