用C语言写一个窗口排队管理系统
时间: 2023-11-06 08:05:43 浏览: 88
好的,下面是一个简单的窗口排队管理系统的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 10
typedef struct {
int num; // 号码
char name[20]; // 姓名
} Window;
typedef struct {
Window data[MAXSIZE]; // 存储窗口信息
int front; // 头指针
int rear; // 尾指针
} Queue;
int InitQueue(Queue *Q) {
Q->front = Q->rear = 0;
return 1;
}
int QueueEmpty(Queue Q) {
if (Q.front == Q.rear) {
return 1;
} else {
return 0;
}
}
int QueueFull(Queue Q) {
if ((Q.rear + 1) % MAXSIZE == Q.front) {
return 1;
} else {
return 0;
}
}
int EnQueue(Queue *Q, Window e) {
if (QueueFull(*Q)) {
return 0;
}
Q->data[Q->rear] = e;
Q->rear = (Q->rear + 1) % MAXSIZE;
return 1;
}
int DeQueue(Queue *Q, Window *e) {
if (QueueEmpty(*Q)) {
return 0;
}
*e = Q->data[Q->front];
Q->front = (Q->front + 1) % MAXSIZE;
return 1;
}
int main() {
Queue Q;
InitQueue(&Q);
int choice, num;
char name[20];
Window e;
while (1) {
printf("请选择操作:\n");
printf("1.新号码进入队列\n");
printf("2.叫号\n");
printf("3.退出\n");
scanf("%d", &choice);
switch (choice) {
case 1:
if (QueueFull(Q)) {
printf("队列已满,无法添加新号码!\n");
} else {
printf("请输入新号码的信息:\n");
printf("号码:");
scanf("%d", &num);
printf("姓名:");
scanf("%s", name);
e.num = num;
strcpy(e.name, name);
EnQueue(&Q, e);
printf("新号码已成功添加到队列中!\n");
}
break;
case 2:
if (QueueEmpty(Q)) {
printf("队列为空,无法叫号!\n");
} else {
DeQueue(&Q, &e);
printf("请 %s (%d) 到 %d 号窗口办理业务!\n", e.name, e.num, Q.front + 1);
}
break;
case 3:
exit(0);
break;
default:
printf("输入有误,请重新输入!\n");
break;
}
}
return 0;
}
```
这个代码示例中,我们定义了一个 Window 结构体来存储窗口信息,定义了一个 Queue 结构体来实现队列的功能。具体实现过程中,我们用 InitQueue 函数来初始化队列,用 QueueEmpty 和 QueueFull 函数来判断队列是否为空或已满,用 EnQueue 函数来将新号码加入队列,用 DeQueue 函数来从队列中叫号。最后,在 main 函数中,根据用户的选择来执行相应的操作。
相关推荐
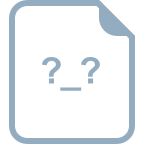
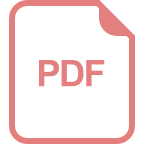
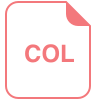
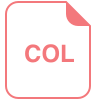
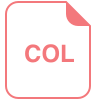
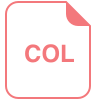
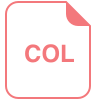







