c语言窗口排队管理系统代码
时间: 2023-08-06 09:03:04 浏览: 93
这是一个简单的C语言窗口排队管理系统的代码,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 20
// 窗口结构体
typedef struct Window {
int win_id; // 窗口号
char win_name[MAX_NAME_LEN]; // 窗口名称
int is_free; // 窗口是否空闲
struct Customer *cur_customer; // 当前服务的顾客
struct Window *next; // 下一个窗口
} Window;
// 顾客结构体
typedef struct Customer {
int cus_id; // 顾客号
char cus_name[MAX_NAME_LEN]; // 顾客姓名
int arrive_time; // 到达时间
int service_time; // 服务时间
struct Customer *next; // 下一个顾客
} Customer;
// 在链表尾部插入窗口
void insert_window(Window **head, int id, char *name) {
Window *new_win = (Window *)malloc(sizeof(Window));
new_win->win_id = id;
strcpy(new_win->win_name, name);
new_win->is_free = 1;
new_win->cur_customer = NULL;
new_win->next = NULL;
if (*head == NULL) {
*head = new_win;
} else {
Window *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = new_win;
}
}
// 在链表尾部插入顾客
void insert_customer(Customer **head, int id, char *name, int arrive_time, int service_time) {
Customer *new_cus = (Customer *)malloc(sizeof(Customer));
new_cus->cus_id = id;
strcpy(new_cus->cus_name, name);
new_cus->arrive_time = arrive_time;
new_cus->service_time = service_time;
new_cus->next = NULL;
if (*head == NULL) {
*head = new_cus;
} else {
Customer *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = new_cus;
}
}
// 根据到达时间排序顾客链表
void sort_customer(Customer **head) {
if (*head == NULL || (*head)->next == NULL) {
return;
}
Customer *p, *q, *temp;
p = *head;
*head = NULL;
while (p != NULL) {
temp = p;
p = p->next;
if (*head == NULL || (*head)->arrive_time > temp->arrive_time) {
temp->next = *head;
*head = temp;
} else {
q = *head;
while (q->next != NULL && q->next->arrive_time <= temp->arrive_time) {
q = q->next;
}
temp->next = q->next;
q->next = temp;
}
}
}
// 根据窗口号查找窗口
Window *find_window_by_id(Window *head, int id) {
Window *p = head;
while (p != NULL && p->win_id != id) {
p = p->next;
}
return p;
}
// 根据顾客号查找顾客
Customer *find_customer_by_id(Customer *head, int id) {
Customer *p = head;
while (p != NULL && p->cus_id != id) {
p = p->next;
}
return p;
}
// 查找空闲窗口
Window *find_free_window(Window *head) {
Window *p = head;
while (p != NULL && p->is_free == 0) {
p = p->next;
}
return p;
}
// 更新窗口状态
void update_window_status(Window *win, Customer *cus) {
win->is_free = 0;
win->cur_customer = cus;
}
// 从链表中删除顾客
void remove_customer(Customer **head, Customer *cus) {
if (*head == cus) {
*head = (*head)->next;
} else {
Customer *p = *head;
while (p != NULL && p->next != cus) {
p = p->next;
}
if (p != NULL) {
p->next = cus->next;
}
}
free(cus);
}
// 从窗口中移除顾客
void remove_customer_from_window(Window *win) {
win->is_free = 1;
win->cur_customer = NULL;
}
// 打印窗口信息
void print_window_info(Window *head) {
Window *p = head;
while (p != NULL) {
printf("Window %d: %s, %s\n", p->win_id, p->win_name, p->is_free ? "Free" : "Busy");
if (!p->is_free) {
printf("\tServing customer: %d, %s\n", p->cur_customer->cus_id, p->cur_customer->cus_name);
}
p = p->next;
}
}
// 打印顾客信息
void print_customer_info(Customer *head) {
Customer *p = head;
while (p != NULL) {
printf("Customer %d: %s, Arrive time: %d, Service time: %d\n", p->cus_id, p->cus_name, p->arrive_time, p->service_time);
p = p->next;
}
}
int main() {
Window *win_head = NULL;
Customer *cus_head = NULL;
// 初始化窗口
insert_window(&win_head, 1, "Window 1");
insert_window(&win_head, 2, "Window 2");
insert_window(&win_head, 3, "Window 3");
insert_window(&win_head, 4, "Window 4");
int choice;
while (1) {
printf("1. Add customer\n");
printf("2. Serve customer\n");
printf("3. Print window info\n");
printf("4. Print customer info\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch(choice) {
case 1: {
int cus_id, arrive_time, service_time;
char cus_name[MAX_NAME_LEN];
printf("Enter customer ID: ");
scanf("%d", &cus_id);
printf("Enter customer name: ");
scanf("%s", cus_name);
printf("Enter arrive time: ");
scanf("%d", &arrive_time);
printf("Enter service time: ");
scanf("%d", &service_time);
insert_customer(&cus_head, cus_id, cus_name, arrive_time, service_time);
sort_customer(&cus_head);
printf("Customer added successfully.\n");
break;
}
case 2: {
int win_id, cus_id;
printf("Enter window ID: ");
scanf("%d", &win_id);
printf("Enter customer ID: ");
scanf("%d", &cus_id);
Window *win = find_window_by_id(win_head, win_id);
Customer *cus = find_customer_by_id(cus_head, cus_id);
if (win == NULL) {
printf("Invalid window ID.\n");
} else if (cus == NULL) {
printf("Invalid customer ID.\n");
} else if (win->is_free == 0) {
printf("Window is busy.\n");
} else {
update_window_status(win, cus);
remove_customer(&cus_head, cus);
printf("Customer %d is served by window %d.\n", cus_id, win_id);
}
break;
}
case 3: {
print_window_info(win_head);
break;
}
case 4: {
print_customer_info(cus_head);
break;
}
case 5: {
exit(0);
}
default: {
printf("Invalid choice.\n");
break;
}
}
}
return 0;
}
```
注意:这只是一个简单的实现,还有很多可以优化的地方。比如可以使用优先队列来管理顾客,可以添加更多的功能,可以使用更加严谨的输入判断等等。
阅读全文
相关推荐
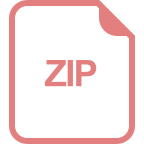
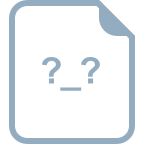
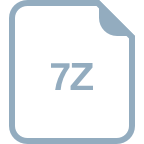















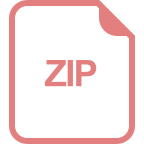