c语言代码银行窗口排队排队管理系统
时间: 2023-07-03 11:30:08 浏览: 134
好的,以下是一个简单的银行窗口排队管理系统的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
// 客户信息结构体
struct customer {
char name[20]; // 客户姓名
int type; // 业务类型
int time; // 办理时间
struct customer *next; // 指向下一个客户的指针
};
// 队列结构体
struct queue {
int count; // 队列中客户数量
struct customer *head; // 队列头指针
struct customer *tail; // 队列尾指针
};
// 初始化队列
void init_queue(struct queue *q) {
q->count = 0;
q->head = NULL;
q->tail = NULL;
}
// 入队操作
void enqueue(struct queue *q, char *name, int type) {
struct customer *new_customer = (struct customer*) malloc(sizeof(struct customer));
strcpy(new_customer->name, name);
new_customer->type = type;
new_customer->time = 0;
new_customer->next = NULL;
if (q->count == 0) {
q->head = new_customer;
q->tail = new_customer;
} else {
q->tail->next = new_customer;
q->tail = new_customer;
}
q->count++;
}
// 出队操作
void dequeue(struct queue *q) {
if (q->count > 0) {
struct customer *customer_to_serve = q->head;
q->head = customer_to_serve->next;
free(customer_to_serve);
q->count--;
}
}
// 显示队列中客户信息
void show_queue(struct queue *q) {
printf("当前排队人数:%d\n", q->count);
printf("姓名\t业务类型\t预计等待时间\n");
struct customer *p = q->head;
int wait_time = 0;
while (p != NULL) {
wait_time += 3; // 假设每个客户办理业务需要3分钟
p->time = wait_time;
printf("%s\t%d\t\t%d\n", p->name, p->type, p->time);
p = p->next;
}
}
int main() {
struct queue q;
init_queue(&q);
while (1) {
// 显示菜单
printf("\n银行业务类型:\n");
printf("1. 存款\n");
printf("2. 取款\n");
printf("3. 转账\n");
printf("4. 查询\n");
printf("0. 退出\n");
// 获取用户输入
int choice;
printf("请输入业务类型:");
scanf("%d", &choice);
if (choice == 0) {
break;
}
// 获取客户姓名
char name[20];
printf("请输入您的姓名:");
scanf("%s", name);
// 将客户加入队列
enqueue(&q, name, choice);
// 显示当前队列中的客户信息
show_queue(&q);
}
return 0;
}
```
这个示例代码实现了银行窗口排队管理系统的基本功能,包括入队、出队、显示队列中客户信息等。当用户输入业务类型和姓名后,客户就会被加入队列中,同时系统会显示当前队列中的客户信息和预计等待时间。可以根据实际需求扩展代码,增加更多的功能。
阅读全文
相关推荐
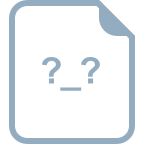

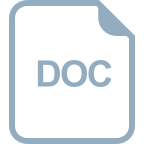










