请用c语言设计并实现一个银行窗口排队管理系统
时间: 2024-02-24 20:55:17 浏览: 27
好的,以下是一个简单的银行窗口排队管理系统的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 10
typedef struct {
int id;
char name[20];
} Customer;
typedef struct {
Customer customer[MAX_QUEUE_SIZE];
int front;
int rear;
} Queue;
void init(Queue *q) {
q->front = q->rear = 0;
}
int isEmpty(Queue *q) {
return q->front == q->rear;
}
int isFull(Queue *q) {
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
void enqueue(Queue *q, Customer c) {
if (isFull(q)) {
printf("Queue is full.\n");
return;
}
q->customer[q->rear] = c;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
Customer dequeue(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
exit(-1);
}
Customer c = q->customer[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return c;
}
void display(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
return;
}
printf("Queue:\n");
for (int i = q->front; i != q->rear; i = (i + 1) % MAX_QUEUE_SIZE) {
printf("\t%d\t%s\n", q->customer[i].id, q->customer[i].name);
}
}
int main() {
Queue q;
init(&q);
int option;
do {
printf("1. Enqueue\n2. Dequeue\n3. Display\n4. Exit\n");
printf("Enter option: ");
scanf("%d", &option);
switch (option) {
case 1: {
Customer c;
printf("Enter customer id: ");
scanf("%d", &c.id);
printf("Enter customer name: ");
scanf("%s", c.name);
enqueue(&q, c);
break;
}
case 2: {
Customer c = dequeue(&q);
printf("Dequeued customer: %d\t%s\n", c.id, c.name);
break;
}
case 3: {
display(&q);
break;
}
case 4: {
printf("Exiting...\n");
break;
}
default: {
printf("Invalid option.\n");
}
}
} while (option != 4);
return 0;
}
```
这个程序中,我们定义了一个 `Customer` 结构体来表示银行排队的顾客,包括顾客的 ID 和名字。我们还定义了一个 `Queue` 结构体来表示队列,包括一个 `customer` 数组和队列的前后指针 `front` 和 `rear`。我们使用循环数组来实现队列的存储,以便队列可以循环利用存储空间。
在 `init` 函数中,我们初始化队列的前后指针。在 `isEmpty` 和 `isFull` 函数中,我们分别判断队列是否为空和是否已满。在 `enqueue` 函数中,我们将顾客加入队列的末尾,如果队列已满则提示错误。在 `dequeue` 函数中,我们将队列头部的顾客取出并返回,如果队列为空则提示错误。在 `display` 函数中,我们遍历队列并输出每个顾客的 ID 和名字。
在主函数中,我们提供了四个选项:加入队列、取出队列、显示队列和退出程序。我们使用 `switch` 语句来处理用户输入的选项,并调用相应的函数。当用户选择退出程序时,我们跳出循环并结束程序。
相关推荐
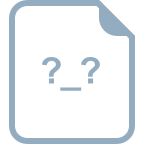














