用C语言设计并实现一个银行窗口排队管理系统,模拟用户排队、办理业务结束后离队等过程,要求窗口数量无限制
时间: 2024-02-28 19:55:05 浏览: 20
好的,我可以为您解答这个问题。银行窗口排队管理系统可以通过使用队列来实现。在C语言中,可以使用结构体来表示队列中的每个元素。
首先,需要定义一个结构体来表示队列中的每个用户。
```
typedef struct {
int id; // 用户ID
int service; // 服务类型
} Customer;
```
其中,id表示用户的编号,service表示用户需要办理的业务类型。
接下来,需要定义一个结构体来表示队列。
```
typedef struct {
Customer *customers; // 存储客户的指针数组
int front; // 队头指针
int rear; // 队尾指针
int size; // 队列当前大小
int capacity; // 队列容量
} Queue;
```
其中,customers是一个指针数组,用于存储队列中的客户。front和rear分别表示队头和队尾的指针。size表示队列当前大小,capacity表示队列容量。
接下来,可以定义一些函数来操作队列。
首先,需要定义一个函数来创建队列。
```
Queue *createQueue(int capacity) {
Queue *queue = (Queue *)malloc(sizeof(Queue));
queue->customers = (Customer *)malloc(capacity * sizeof(Customer));
queue->front = 0;
queue->rear = -1;
queue->size = 0;
queue->capacity = capacity;
return queue;
}
```
这个函数会创建一个指定容量的队列,并返回一个指向该队列的指针。
接下来,需要定义一个函数来判断队列是否为空。
```
int isEmpty(Queue *queue) {
return queue->size == 0;
}
```
这个函数会返回一个布尔值,用于判断队列是否为空。
接下来,需要定义一个函数来判断队列是否已满。
```
int isFull(Queue *queue) {
return queue->size == queue->capacity;
}
```
这个函数会返回一个布尔值,用于判断队列是否已满。
接下来,需要定义一个函数来向队列中添加客户。
```
void enqueue(Queue *queue, Customer customer) {
if (isFull(queue)) {
printf("Queue is full\n");
return;
}
queue->rear = (queue->rear + 1) % queue->capacity;
queue->customers[queue->rear] = customer;
queue->size++;
}
```
这个函数会向队列中添加一个客户。如果队列已满,则会输出一条错误信息。
接下来,需要定义一个函数来从队列中删除客户。
```
Customer dequeue(Queue *queue) {
if (isEmpty(queue)) {
printf("Queue is empty\n");
Customer customer = {-1, -1};
return customer;
}
Customer customer = queue->customers[queue->front];
queue->front = (queue->front + 1) % queue->capacity;
queue->size--;
return customer;
}
```
这个函数会从队列中删除一个客户,并返回该客户的信息。如果队列为空,则会输出一条错误信息,并返回一个ID为-1的客户。
最后,可以编写一个主函数来模拟银行窗口排队管理系统。
```
int main() {
Queue *queue = createQueue(100);
int customerId = 0;
while (1) {
// 模拟用户排队
Customer customer = {customerId++, rand() % 3};
printf("Customer %d enters the queue\n", customer.id);
enqueue(queue, customer);
// 模拟窗口服务
if (!isEmpty(queue)) {
Customer customer = dequeue(queue);
printf("Customer %d leaves the queue\n", customer.id);
}
// 模拟用户离队
if (customerId == 10) {
break;
}
}
return 0;
}
```
这个主函数会模拟用户排队、窗口服务和用户离队等过程。其中,每个用户的服务类型是随机的,有三种可能性。在服务过程中,会从队列中删除一个客户,并输出该客户的信息。当10个用户都离开队列时,程序会结束。
希望这个银行窗口排队管理系统的实现对您有所帮助!
相关推荐
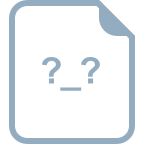














