用c++编写程序题目:办公室日常管理信息系统 系统功能基本要求: 文件管理信息:包括文件编号、文件种类、文件名称、存放位置等; 考勤管理:包括姓名、年龄、职务、日期、出勤情况等;查询员工的出勤情况。会议记录:包括会议时间、参会人、记录员、会议内容等; 办公室日常事务管理,包括时间、事务、记录人。按条件查询,统计(用文件存储信息)
时间: 2023-07-10 12:34:44 浏览: 83
好的,这个问题需要编写一个办公室日常管理信息系统,涵盖文件管理、考勤管理、会议记录和办公室日常事务管理四个模块,并且可以按条件查询和统计数据。下面是一个简单的 C++ 代码示例,可以作为您编写程序的参考:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 文件结构体
struct File {
int id; // 文件编号
string type; // 文件种类
string name; // 文件名称
string location; // 存放位置
};
// 员工结构体
struct Employee {
string name; // 姓名
int age; // 年龄
string position; // 职务
vector<string> attendance; // 出勤情况
};
// 会议结构体
struct Meeting {
string time; // 会议时间
vector<string> participants; // 参会人
string recorder; // 记录员
string content; // 会议内容
};
// 办公室事务结构体
struct Task {
string time; // 时间
string content; // 事务内容
string recorder; // 记录人
};
// 文件管理类
class FileManager {
public:
FileManager(const string& file_name) {
this->file_name = file_name;
}
void add_file(const File& file) {
files.push_back(file);
}
void remove_file(int file_id) {
for (auto it = files.begin(); it != files.end(); ++it) {
if (it->id == file_id) {
files.erase(it);
break;
}
}
}
void save_to_file() {
ofstream output_file(file_name);
for (const auto& file : files) {
output_file << file.id << "," << file.type << "," << file.name << "," << file.location << endl;
}
output_file.close();
}
void load_from_file() {
files.clear();
ifstream input_file(file_name);
string line;
while (getline(input_file, line)) {
int id = stoi(line.substr(0, line.find(',')));
line = line.substr(line.find(',') + 1);
string type = line.substr(0, line.find(','));
line = line.substr(line.find(',') + 1);
string name = line.substr(0, line.find(','));
line = line.substr(line.find(',') + 1);
string location = line;
files.push_back({id, type, name, location});
}
input_file.close();
}
vector<File> search_by_type(const string& type) {
vector<File> result;
for (const auto& file : files) {
if (file.type == type) {
result.push_back(file);
}
}
return result;
}
vector<File> search_by_name(const string& name) {
vector<File> result;
for (const auto& file : files) {
if (file.name == name) {
result.push_back(file);
}
}
return result;
}
vector<File> search_by_location(const string& location) {
vector<File> result;
for (const auto& file : files) {
if (file.location == location) {
result.push_back(file);
}
}
return result;
}
private:
string file_name; // 文件名
vector<File> files; // 文件列表
};
// 考勤管理类
class AttendanceManager {
public:
AttendanceManager(const string& file_name) {
this->file_name = file_name;
}
void add_employee(const Employee& employee) {
employees.push_back(employee);
}
void remove_employee(const string& name) {
for (auto it = employees.begin(); it != employees.end(); ++it) {
if (it->name == name) {
employees.erase(it);
break;
}
}
}
void save_to_file() {
ofstream output_file(file_name);
for (const auto& employee : employees) {
output_file << employee.name << "," << employee.age << "," << employee.position << ",";
for (const auto& attendance : employee.attendance) {
output_file << attendance << ",";
}
output_file << endl;
}
output_file.close();
}
void load_from_file() {
employees.clear();
ifstream input_file(file_name);
string line;
while (getline(input_file, line)) {
string name = line.substr(0, line.find(','));
line = line.substr(line.find(',') + 1);
int age = stoi(line.substr(0, line.find(',')));
line = line.substr(line.find(',') + 1);
string position = line.substr(0, line.find(','));
line = line.substr(line.find(',') + 1);
vector<string> attendance;
while (!line.empty()) {
attendance.push_back(line.substr(0, line.find(',')));
line = line.substr(line.find(',') + 1);
}
employees.push_back({name, age, position, attendance});
}
input_file.close();
}
vector<Employee> search_by_name(const string& name) {
vector<Employee> result;
for (const auto& employee : employees) {
if (employee.name == name) {
result.push_back(employee);
}
}
return result;
}
void set_attendance(const string& name, const string& date, const string& status) {
for (auto& employee : employees) {
if (employee.name == name) {
for (auto& attendance : employee.attendance) {
if (attendance.substr(0, attendance.find(' ')) == date) {
attendance = date + " " + status;
return;
}
}
employee.attendance.push_back(date + " " + status);
return;
}
}
}
private:
string file_name; // 文件名
vector<Employee> employees; // 员工列表
};
// 会议记录管理类
class MeetingManager {
public:
MeetingManager(const string& file_name) {
this->file_name = file_name;
}
void add_meeting(const Meeting& meeting) {
meetings.push_back(meeting);
}
void remove_meeting(const string& time) {
for (auto it = meetings.begin(); it != meetings.end(); ++it) {
if (it->time == time) {
meetings.erase(it);
break;
}
}
}
void save_to_file() {
ofstream output_file(file_name);
for (const auto& meeting : meetings) {
output_file << meeting.time << ",";
for (const auto& participant : meeting.participants) {
output_file << participant << ",";
}
output_file << meeting.recorder << "," << meeting.content << endl;
}
output_file.close();
}
void load_from_file() {
meetings.clear();
ifstream input_file(file_name);
string line;
while (getline(input_file, line)) {
string time = line.substr(0, line.find(','));
line = line.substr(line.find(',') + 1);
vector<string> participants;
while (!line.empty() && line[0] != ',') {
participants.push_back(line.substr(0, line.find(',')));
line = line.substr(line.find(',') + 1);
}
string recorder = line.substr(1, line.find(',') - 1);
line = line.substr(line.find(',') + 1);
string content = line;
meetings.push_back({time, participants, recorder, content});
}
input_file.close();
}
vector<Meeting> search_by_participant(const string& name) {
vector<Meeting> result;
for (const auto& meeting : meetings) {
if (find(meeting.participants.begin(), meeting.participants.end(), name) != meeting.participants.end()) {
result.push_back(meeting);
}
}
return result;
}
private:
string file_name; // 文件名
vector<Meeting> meetings; // 会议列表
};
// 办公室事务管理类
class TaskManager {
public:
TaskManager(const string& file_name) {
this->file_name = file_name;
}
void add_task(const Task& task) {
tasks.push_back(task);
}
void remove_task(const string& time) {
for (auto it = tasks.begin(); it != tasks.end(); ++it) {
if (it->time == time) {
tasks.erase(it);
break;
}
}
}
void save_to_file() {
ofstream output_file(file_name);
for (const auto& task : tasks) {
output_file << task.time << "," << task.content << "," << task.recorder << endl;
}
output_file.close();
}
void load_from_file() {
tasks.clear();
ifstream input_file(file_name);
string line;
while (getline(input_file, line)) {
string time = line.substr(0, line.find(','));
line = line.substr(line.find(',') + 1);
string content = line.substr(0, line.find(','));
line = line.substr(line.find(',') + 1);
string recorder = line;
tasks.push_back({time, content, recorder});
}
input_file.close();
}
vector<Task> search_by_time(const string& time) {
vector<Task> result;
for (const auto& task : tasks) {
if (task.time == time) {
result.push_back(task);
}
}
return result;
}
private:
string file_name; // 文件名
vector<Task> tasks; // 事务列表
};
int main() {
FileManager file_manager("files.txt");
file_manager.load_from_file();
file_manager.add_file({1, "文档", "公司章程", "A101"});
file_manager.add_file({2, "表格", "财务报表", "A102"});
file_manager.add_file({3, "图片", "公司logo", "A103"});
file_manager.save_to_file();
auto files = file_manager.search_by_type("文档");
for (const auto& file : files) {
cout << file.id << " " << file.type << " " << file.name << " " << file.location << endl;
}
AttendanceManager attendance_manager("attendance.txt");
attendance_manager.load_from_file();
attendance_manager.add_employee({"张三", 30, "经理", {"2022-01-01 上班", "2022-01-02 上班", "2022-01-03 请假"}});
attendance_manager.add_employee({"李四", 25, "职员", {"2022-01-01 上班", "2022-01-02 请假", "2022-01-03 上班"}});
attendance_manager.set_attendance("李四", "2022-01-02", "迟到");
attendance_manager.save_to_file();
auto employees = attendance_manager.search_by_name("李四");
for (const auto& employee : employees) {
cout << employee.name << " " << employee.age << " " << employee.position << " ";
for (const auto& attendance : employee.attendance) {
cout << attendance << " ";
}
cout << endl;
}
MeetingManager meeting_manager("meetings.txt");
meeting_manager.load_from_file();
meeting_manager.add_meeting({"2022-01-01 10:00", {"张三", "李四", "王五"}, "赵六", "讨论2022年工作计划"});
meeting_manager.add_meeting({"2022-01-02 14:00", {"赵六", "钱七", "孙八"}, "李四", "讨论2022年财务计划"});
meeting_manager.remove_meeting("2022-01-01 10:00");
meeting_manager.save_to_file();
auto meetings = meeting_manager.search_by_participant("赵六");
for (const auto& meeting : meetings) {
cout << meeting.time << " ";
for (const auto& participant : meeting.participants) {
cout << participant << " ";
}
cout << meeting.recorder << " " << meeting.content << endl;
}
TaskManager task_manager("tasks.txt");
task_manager.load_from_file();
task_manager.add_task({"2022-01-02 09:00", "开会", "张三"});
task_manager.add_task({"2022-01-03 14:00", "提交工作报告", "李四"});
task_manager.remove_task("2022-01-02 09:00");
task_manager.save_to_file();
auto tasks = task_manager.search_by_time("2022-01-03 14:00");
for (const auto& task : tasks) {
cout << task.time << " " << task.content << " " << task.recorder << endl;
}
return 0;
}
```
这个程序使用了四个类来管理文件、考勤、会议和办公室事务四个模块。每个类都有自己的数据结构和方法,可以进行增删改查等操作。在程序运行时,先从文件中读取数据,然后进行操作后再将数据写回文件中。您可以根据自己的需求进行修改和扩展。
相关推荐
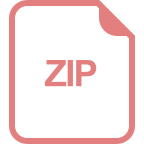
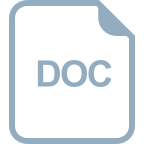
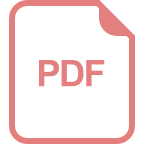


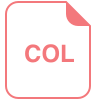
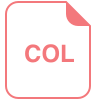
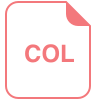









