设计个绘制不同图形的类 能改变图形颜色和线宽
时间: 2023-11-27 22:49:30 浏览: 58
好的,以下是一个基本的类设计:
```python
class Shape:
def __init__(self, color, line_width):
self.color = color
self.line_width = line_width
def draw(self):
pass
class Circle(Shape):
def __init__(self, color, line_width, radius):
super().__init__(color, line_width)
self.radius = radius
def draw(self):
print(f"Drawing a circle with color {self.color} and line width {self.line_width} and radius {self.radius}")
class Rectangle(Shape):
def __init__(self, color, line_width, width, height):
super().__init__(color, line_width)
self.width = width
self.height = height
def draw(self):
print(f"Drawing a rectangle with color {self.color} and line width {self.line_width} and width {self.width} and height {self.height}")
```
在这个类设计中,我们有一个基本的 `Shape` 类,它有两个属性:颜色和线宽。它还有一个 `draw()` 方法,但是它是一个空方法。这是因为我们无法画一个通用的形状,所以我们需要子类来实现它。
我们还有两个子类:`Circle` 和 `Rectangle`。它们都继承了 `Shape`,并添加了它们自己的属性和 `draw()` 方法。注意,在子类的构造函数中,我们使用 `super().__init__()` 来调用父类的构造函数,以便我们可以设置颜色和线宽。
现在我们可以使用这些类来创建和绘制不同的图形,例如:
```python
red_circle = Circle("red", 2, 5)
red_circle.draw()
blue_rectangle = Rectangle("blue", 1, 3, 4)
blue_rectangle.draw()
```
这将输出:
```
Drawing a circle with color red and line width 2 and radius 5
Drawing a rectangle with color blue and line width 1 and width 3 and height 4
```
我们可以看到,我们可以创建不同颜色、线宽和大小的圆和矩形,并使用它们的 `draw()` 方法来绘制它们。
阅读全文
相关推荐
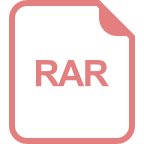
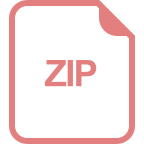
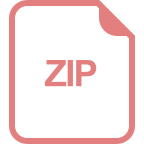
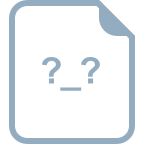
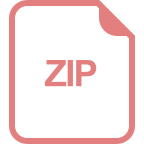
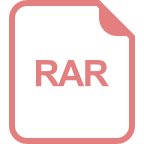
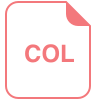






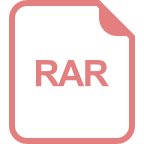
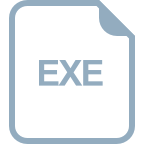
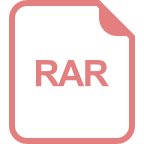