请帮我写python解决八数码问题,使用广度优先算法
时间: 2023-06-02 18:08:16 浏览: 156
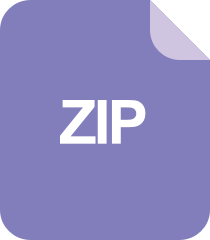
基于Python的多种算法实现八数码问题求解设计源码
好的,我可以帮你解决八数码问题!以下是Python代码:
```python
# 定义初始状态和目标状态
start_state = [2, 8, 3, 1, 6, 4, 7, 0, 5]
goal_state = [1, 2, 3, 8, 0, 4, 7, 6, 5]
# 定义移动函数
def move(state, direction):
new_state = state.copy()
index = new_state.index(0)
if direction == 'left':
if index not in [0, 3, 6]:
new_state[index], new_state[index-1] = new_state[index-1], new_state[index]
return new_state
else:
return None
if direction == 'right':
if index not in [2, 5, 8]:
new_state[index], new_state[index+1] = new_state[index+1], new_state[index]
return new_state
else:
return None
if direction == 'up':
if index not in [0, 1, 2]:
new_state[index], new_state[index-3] = new_state[index-3], new_state[index]
return new_state
else:
return None
if direction == 'down':
if index not in [6, 7, 8]:
new_state[index], new_state[index+3] = new_state[index+3], new_state[index]
return new_state
else:
return None
# 定义广度优先搜索函数
def bfs(start, goal):
queue = [[start]]
visited = set()
while queue:
path = queue.pop(0)
node = path[-1]
if node == goal:
return path
if tuple(node) not in visited:
visited.add(tuple(node))
for direction in ['up', 'down', 'left', 'right']:
child = move(node, direction)
if child is not None:
new_path = list(path)
new_path.append(child)
queue.append(new_path)
print(bfs(start_state, goal_state))
```
该代码使用广度优先搜索算法解决八数码问题。通过将当前状态扩展成所有可能的下一步状态,不断搜索直到找到目标状态。注意,为了避免进入无限循环,我们使用了一个“visited”集合来跟踪已访问的状态,以及一个“path”列表来记录搜索路径。
阅读全文
相关推荐
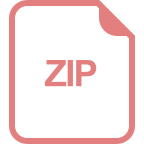
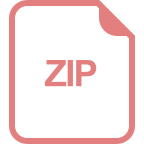















