7-2 数据结构考题 二叉树的遍历-中序
时间: 2023-09-13 12:09:17 浏览: 197
二叉树的中序遍历是一种常见的遍历方式,遵循左子树-根节点-右子树的顺序。具体的实现可以使用递归或者迭代的方式。
递归实现中序遍历的代码如下:
```python
def inorderTraversal(root):
result = []
inorderHelper(root, result)
return result
def inorderHelper(node, result):
if node is None:
return
inorderHelper(node.left, result)
result.append(node.val)
inorderHelper(node.right, result)
```
迭代实现中序遍历的代码如下:
```python
def inorderTraversal(root):
result = []
stack = []
curr = root
while curr is not None or stack:
while curr is not None:
stack.append(curr)
curr = curr.left
curr = stack.pop()
result.append(curr.val)
curr = curr.right
return result
```
以上代码中,我们使用一个栈来辅助迭代实现中序遍历。首先将根节点入栈,然后将左子树一直入栈直到为空。然后弹出栈顶元素,将其值加入结果列表,并将当前节点指向右子树。重复上述操作直到栈为空且当前节点为空。
希望以上代码可以帮助到你!如果还有其他问题,请继续提问。
阅读全文
相关推荐
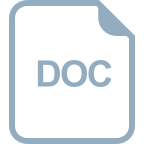
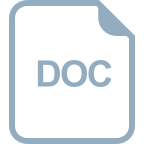
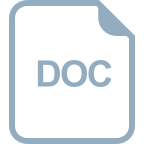
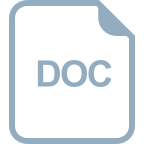
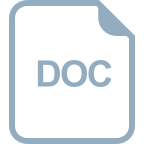
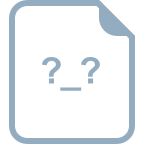
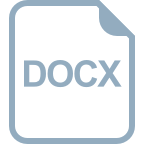
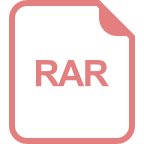
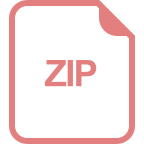
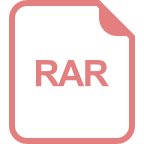
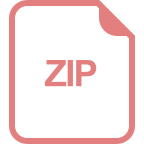
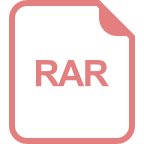
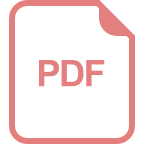
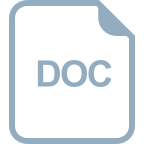
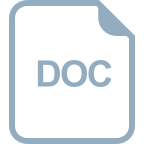
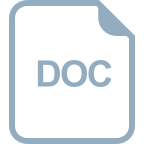
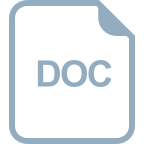
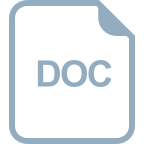
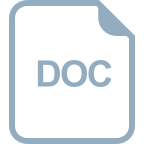