typescript删除数组空字符串
时间: 2023-09-30 10:05:36 浏览: 152
可以使用Array.filter()方法来删除数组中的空字符串。
示例代码:
```typescript
const arr: string[] = ['foo', '', 'bar', ' ', 'baz', ''];
const filteredArr = arr.filter((value) => value.trim() !== '');
console.log(filteredArr); // ['foo', 'bar', 'baz']
```
在上面的代码中,我们使用Array.filter()方法来创建一个新的数组,其中仅包含非空字符串。在回调函数中,我们使用String.trim()方法删除字符串前后的空格,并检查字符串是否为空字符串。如果字符串不为空,则返回true,否则返回false。只有返回true的元素才会被包含在新数组中。
相关问题
typescript 数组
TypeScript中的数组是一种特殊的数据类型,可以存储多个相同或不同类型的元素。你可以使用以下方式声明和操作数组:
1. 声明一个数组变量:
```typescript
let myArray: number[]; // 声明一个名为myArray的数字数组
```
2. 初始化一个数组:
```typescript
let myArray: number[] = [1, 2, 3]; // 初始化一个包含数字的数组
let myArray: Array<string> = ["apple", "banana", "orange"]; // 使用泛型声明一个包含字符串的数组
```
3. 访问和修改数组元素:
```typescript
let myArray: number[] = [1, 2, 3];
console.log(myArray[0]); // 输出第一个元素:1
myArray[1] = 5; // 修改第二个元素为5
```
4. 数组方法:
TypeScript提供了许多内置数组方法来操作和处理数组。一些常用的方法如下:
```typescript
let myArray: number[] = [1, 2, 3];
myArray.push(4); // 向数组末尾添加元素
myArray.pop(); // 删除并返回数组末尾的元素
myArray.length; // 获取数组的长度
myArray.concat([5, 6]); // 连接两个数组
myArray.slice(1, 3); // 返回从索引1到索引3(不包括索引3)的子数组
myArray.splice(1, 2); // 删除从索引1开始的2个元素,并返回被删除的元素
myArray.forEach((element) => {
console.log(element); // 遍历数组并输出每个元素
});
```
这些只是一些基本的操作,TypeScript还提供了更多高级的数组操作方法。你可以根据自己的需求选择合适的方法来操作数组。
第一个参数可以是字符串,也可以是obj数组
好的,这里是一个可以接受字符串或对象作为参数的弹窗方法:
```typescript
interface PopupOptions {
title: string;
content: string;
method: string | (() => void);
}
function popup(options: PopupOptions | string, content?: string, method?: string | (() => void)): void {
let title = '';
let popupContent = '';
let popupMethod: string | (() => void) = '';
if (typeof options === 'string') {
title = options;
popupContent = content || '';
popupMethod = method || '';
} else {
title = options.title;
popupContent = options.content;
popupMethod = options.method;
}
const confirmMethod = typeof popupMethod === 'string' ? window[popupMethod] : popupMethod;
// 创建弹窗 DOM
const popupContainer = document.createElement('div');
popupContainer.classList.add('popup-container');
const popupTitle = document.createElement('h2');
popupTitle.textContent = title;
const contentElement = document.createElement('p');
contentElement.textContent = popupContent;
const confirmButton = document.createElement('button');
confirmButton.textContent = '确认';
confirmButton.addEventListener('click', () => {
confirmMethod();
popupContainer.remove();
});
const cancelButton = document.createElement('button');
cancelButton.textContent = '取消';
cancelButton.addEventListener('click', () => {
popupContainer.remove();
});
const buttonContainer = document.createElement('div');
buttonContainer.classList.add('button-container');
buttonContainer.appendChild(cancelButton);
buttonContainer.appendChild(confirmButton);
popupContainer.appendChild(popupTitle);
popupContainer.appendChild(contentElement);
popupContainer.appendChild(buttonContainer);
document.body.appendChild(popupContainer);
}
```
这个弹窗方法可以接受两种形式的参数:
- 一个字符串作为标题,另外两个字符串分别作为内容和方法名:
```typescript
popup('提示', '确定要删除吗?', 'deleteItem');
```
- 一个包含 `title`、`content` 和 `method` 属性的对象:
```typescript
popup({
title: '提示',
content: '确定要删除吗?',
method: 'deleteItem'
});
```
希望这可以满足您的需求。如果还有任何问题,请随时让我知道。
阅读全文
相关推荐
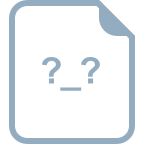
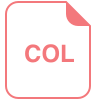
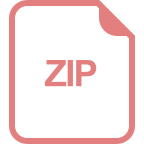
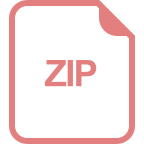
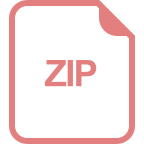
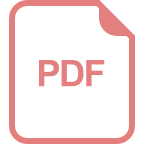
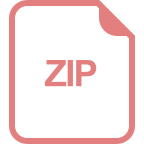
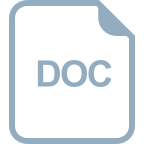
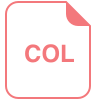
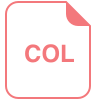
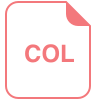
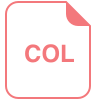


