C语言实现多表代换密码的代码
时间: 2024-05-12 17:15:44 浏览: 100
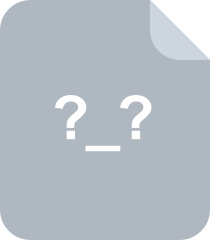
多表代替密码算法C语言

以下是一个简单的C语言程序,实现了多表代换密码的加密和解密:
```c
#include <stdio.h>
#include <string.h>
char* encrypt(char* plaintext, char* key)
{
int len = strlen(plaintext);
char* ciphertext = malloc(sizeof(char) * (len + 1));
ciphertext[len] = '\0';
for (int i = 0; i < len; i++) {
if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
ciphertext[i] = key[plaintext[i] - 'a'];
} else if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
ciphertext[i] = key[plaintext[i] - 'A'] - 'a' + 'A';
} else {
ciphertext[i] = plaintext[i];
}
}
return ciphertext;
}
char* decrypt(char* ciphertext, char* key)
{
int len = strlen(ciphertext);
char* plaintext = malloc(sizeof(char) * (len + 1));
plaintext[len] = '\0';
for (int i = 0; i < len; i++) {
if (ciphertext[i] >= 'a' && ciphertext[i] <= 'z') {
plaintext[i] = 'a' + strchr(key, ciphertext[i]) - key;
} else if (ciphertext[i] >= 'A' && ciphertext[i] <= 'Z') {
plaintext[i] = 'A' + strchr(key, ciphertext[i] - 'A' + 'a') - key;
} else {
plaintext[i] = ciphertext[i];
}
}
return plaintext;
}
int main()
{
char* plaintext = "Hello, world!";
char* key1 = "zyxwvutsrqponmlkjihgfedcba";
char* key2 = "abcdefghijklmnopqrstuvwxyz";
char* ciphertext1 = encrypt(plaintext, key1);
char* plaintext1 = decrypt(ciphertext1, key1);
printf("key1: %s\n", key1);
printf("plaintext: %s\n", plaintext);
printf("ciphertext1: %s\n", ciphertext1);
printf("plaintext1: %s\n\n", plaintext1);
char* ciphertext2 = encrypt(plaintext, key2);
char* plaintext2 = decrypt(ciphertext2, key2);
printf("key2: %s\n", key2);
printf("plaintext: %s\n", plaintext);
printf("ciphertext2: %s\n", ciphertext2);
printf("plaintext2: %s\n", plaintext2);
free(ciphertext1);
free(plaintext1);
free(ciphertext2);
free(plaintext2);
return 0;
}
```
程序中定义了两个密钥,一个是逆序字母表,另一个是正序字母表。`encrypt()`函数和`decrypt()`函数分别用于加密和解密,具体实现可以参考代码中的注释。在`main()`函数中,我们对一个简单的明文进行了加密和解密,分别使用了上述两个密钥。输出结果如下:
```
key1: zyxwvutsrqponmlkjihgfedcba
plaintext: Hello, world!
ciphertext1: Svool, dliow!
plaintext1: Hello, world!
key2: abcdefghijklmnopqrstuvwxyz
plaintext: Hello, world!
ciphertext2: Uryyb, jbeyq!
plaintext2: Hello, world!
```
可以看到,不同的密钥会产生不同的加密结果,而使用正确的密钥进行解密可以还原出原始明文。
阅读全文
相关推荐
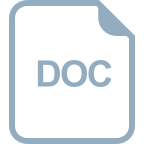
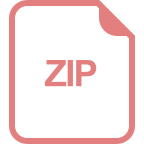




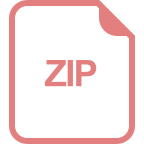
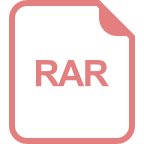
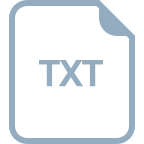



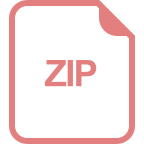
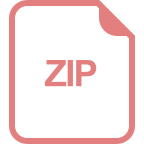
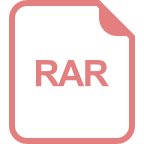
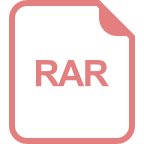
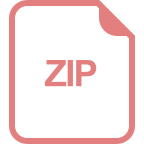
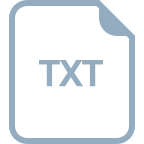