多表代换密码c语言密码学
时间: 2023-06-15 16:06:03 浏览: 254
多表代换密码是一种替代加密技术,它将明文中的每个字母都替换成密文字母。可以使用C语言来实现这种密码。
以下是一个简单的多表代换密码的C语言代码示例:
```c
#include <stdio.h>
#include <string.h>
// 定义密钥表
char table[26] = {'Q', 'W', 'E', 'R', 'T', 'Y', 'U', 'I', 'O', 'P', 'A', 'S', 'D', 'F', 'G', 'H', 'J', 'K', 'L', 'Z', 'X', 'C', 'V', 'B', 'N', 'M'};
// 加密函数
void encrypt(char *plaintext, char *ciphertext) {
int len = strlen(plaintext);
for (int i = 0; i < len; i++) {
if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
ciphertext[i] = table[plaintext[i] - 'a'];
}
else if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
ciphertext[i] = table[plaintext[i] - 'A'];
}
else {
ciphertext[i] = plaintext[i];
}
}
ciphertext[len] = '\0';
}
// 解密函数
void decrypt(char *ciphertext, char *plaintext) {
int len = strlen(ciphertext);
for (int i = 0; i < len; i++) {
if (ciphertext[i] >= 'a' && ciphertext[i] <= 'z') {
for (int j = 0; j < 26; j++) {
if (table[j] == ciphertext[i]) {
plaintext[i] = 'a' + j;
break;
}
}
}
else if (ciphertext[i] >= 'A' && ciphertext[i] <= 'Z') {
for (int j = 0; j < 26; j++) {
if (table[j] == ciphertext[i]) {
plaintext[i] = 'A' + j;
break;
}
}
}
else {
plaintext[i] = ciphertext[i];
}
}
plaintext[len] = '\0';
}
int main() {
char plaintext[100];
char ciphertext[100];
char decryptedtext[100];
printf("请输入明文:");
fgets(plaintext, 100, stdin);
encrypt(plaintext, ciphertext);
printf("加密后的密文:%s\n", ciphertext);
decrypt(ciphertext, decryptedtext);
printf("解密后的明文:%s\n", decryptedtext);
return 0;
}
```
在这个例子中,我们定义了一个密钥表,它包含了26个字母的替代字母。加密函数接收明文作为输入,并将其转换为密文。解密函数接收密文作为输入,并将其转换回明文。最后,我们在主函数中使用这些函数来加密和解密一段文字。
阅读全文
相关推荐
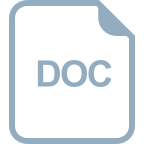
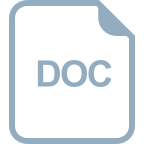
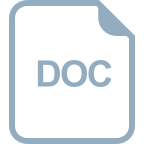
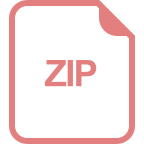
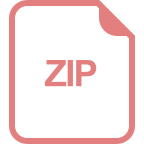

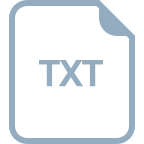
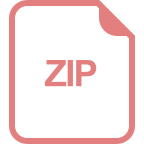
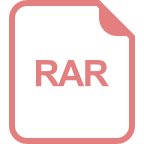
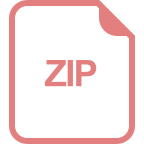
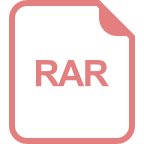
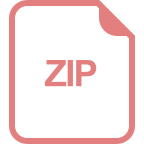
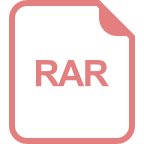
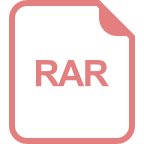
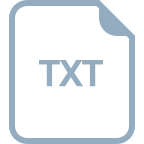
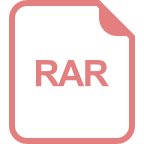
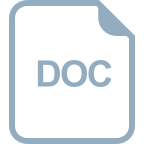
