单表代换密码c语言实现
时间: 2024-05-26 22:06:51 浏览: 113
单表代换密码是一种简单的密码算法,它通过将明文中的每个字母替换为一个固定的字母来进行加密。下面是一个使用C语言实现单表代换密码的例子[^2]:
```c
#include <stdio.h>
#include <string.h>
void encrypt(char *plaintext, char *ciphertext, char *key) {
int i;
int len = strlen(plaintext);
for (i = 0; i < len; i++) {
if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
ciphertext[i] = key[plaintext[i] - 'a'];
} else if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
ciphertext[i] = key[plaintext[i] - 'A' + 26];
} else {
ciphertext[i] = plaintext[i];
}
}
ciphertext[i] = '\0';
}
void decrypt(char *ciphertext, char *plaintext, char *key) {
int i;
int len = strlen(ciphertext);
for (i = 0; i < len; i++) {
if (ciphertext[i] >= 'a' && ciphertext[i] <= 'z') {
plaintext[i] = 'a' + strchr(key, ciphertext[i]) - key;
} else if (ciphertext[i] >= 'A' && ciphertext[i] <= 'Z') {
plaintext[i] = 'A' + strchr(key + 26, ciphertext[i]) - key - 26;
} else {
plaintext[i] = ciphertext[i];
}
}
plaintext[i] = '\0';
}
int main() {
char plaintext[] = "Hello, World!";
char ciphertext[100];
char decrypted[100];
char key[] = "QWERTYUIOPASDFGHJKLZXCVBNMqwertyuiopasdfghjklzxcvbnm";
encrypt(plaintext, ciphertext, key);
printf("Encrypted text: %s\n", ciphertext);
decrypt(ciphertext, decrypted, key);
printf("Decrypted text: %s\n", decrypted);
return 0;
}
```
这段代码中,我们定义了两个函数`encrypt`和`decrypt`来进行加密和解密操作。`encrypt`函数将明文中的每个字母根据密钥替换为对应的字母,`decrypt`函数则将密文中的每个字母根据密钥进行逆向替换。在`main`函数中,我们定义了一个明文字符串`plaintext`,并使用密钥`key`对其进行加密和解密操作。最后,我们打印出加密后的密文和解密后的明文。
阅读全文
相关推荐




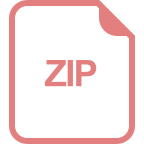
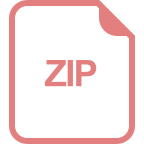
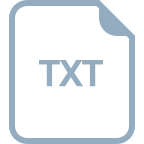
