用c语言实现多表代换密码
时间: 2023-06-15 17:05:57 浏览: 156
多表代换密码是一种基于替换的密码算法,它将明文字符替换为密文字符,从而实现加密。在多表代换密码中,通常采用多个替换表来进行加密,以增加密码的复杂度和安全性。
下面是一个简单的用 C 语言实现多表代换密码的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TABLES 26
#define TABLE_SIZE 26
// 生成随机的替换表
void generate_tables(char tables[MAX_TABLES][TABLE_SIZE+1])
{
int i, j;
for (i = 0; i < MAX_TABLES; i++) {
char c = 'a' + i; // 生成替换表的第一个字符
tables[i][0] = c;
for (j = 1; j < TABLE_SIZE; j++) {
tables[i][j] = c + j;
if (tables[i][j] > 'z') {
tables[i][j] -= 26;
}
}
tables[i][TABLE_SIZE] = '\0';
}
}
// 加密函数
char* encrypt(const char* plain, const char tables[MAX_TABLES][TABLE_SIZE+1])
{
int len = strlen(plain);
char* cipher = (char*) malloc(len+1);
int i, j;
for (i = 0; i < len; i++) {
char c = plain[i];
if (c >= 'a' && c <= 'z') {
int table = c - 'a'; // 根据明文字符选择替换表
j = rand() % TABLE_SIZE; // 随机选择替换字符
cipher[i] = tables[table][j];
} else {
cipher[i] = c;
}
}
cipher[len] = '\0';
return cipher;
}
// 解密函数
char* decrypt(const char* cipher, const char tables[MAX_TABLES][TABLE_SIZE+1])
{
int len = strlen(cipher);
char* plain = (char*) malloc(len+1);
int i, j;
for (i = 0; i < len; i++) {
char c = cipher[i];
if (c >= 'a' && c <= 'z') {
int table = c - 'a'; // 根据密文字符选择替换表
j = strcspn(tables[table], &cipher[i]); // 查找替换字符在替换表中的位置
plain[i] = 'a' + j; // 明文字符为替换字符在替换表中的位置加上 'a'
} else {
plain[i] = c;
}
}
plain[len] = '\0';
return plain;
}
int main()
{
char tables[MAX_TABLES][TABLE_SIZE+1];
generate_tables(tables);
const char* plain = "hello world";
printf("Plain text: %s\n", plain);
char* cipher = encrypt(plain, tables);
printf("Cipher text: %s\n", cipher);
char* decrypted = decrypt(cipher, tables);
printf("Decrypted text: %s\n", decrypted);
free(cipher);
free(decrypted);
return 0;
}
```
在这个示例中,我们首先生成了 26 个大小为 26 的随机替换表,然后使用 `encrypt()` 函数对明文进行加密,使用 `decrypt()` 函数对密文进行解密。在加密过程中,对于明文中的每个小写字母,我们根据它在字母表中的位置选择一个替换表,然后随机选择一个替换字符进行替换。在解密过程中,对于密文中的每个小写字母,我们根据它所代表的替换表和替换字符进行逆替换,得到明文字符。
需要注意的是,这个示例中的多表代换密码算法并不是非常安全,因为替换表是固定的,而且对于明文中的每个小写字母都使用了不同的替换表,这使得攻击者可以通过分析密文中的字母频率来破译密码。实际应用中,应该使用更加复杂的算法来生成替换表,以及使用更加灵活的方法来选择替换字符和替换表。
相关推荐
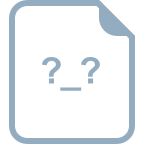
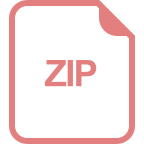
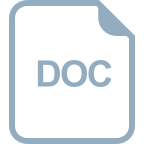








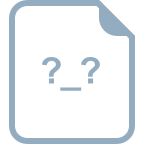
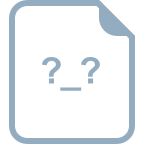