多表代换密码用c语言实现
时间: 2024-09-09 20:11:29 浏览: 60
多表代换密码是一种简单的替换加密技术,它通过将明文字符替换到密钥表中的对应位置来进行加密。在C语言中实现这种密码的一个基本步骤如下:
1. **定义密钥表**:创建两个大小相同的字符数组,一个存储原始字母表(比如小写字母),另一个存储加密后的字母表(可以随机生成或预设密钥)。
```c
#include <stdio.h>
#include <string.h>
char plaintext[26] = {'a', 'b', 'c', ..., 'z'};
char ciphertext[26];
```
2. **加密函数**:接收明文字符串和密钥表作为输入,遍历每个字符,查找其在plaintext数组中的位置,并替换为ciphertext数组中的相应位置。
```c
void encrypt(char *input, char *output, char *key) {
for (int i = 0; input[i] != '\0'; i++) {
int index = input[i] - 'a';
output[i] = key[index];
}
}
```
3. **示例使用**:调用encrypt函数并提供明文和密钥表。
```c
void main() {
char plain_text[] = "hello world";
encrypt(plain_text, ciphertext, plaintext);
// 输出加密后的结果
printf("Encrypted text: %s\n", ciphertext);
}
```
阅读全文
相关推荐
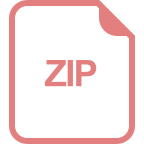
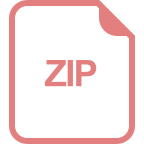
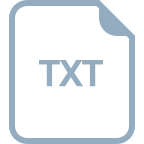











