使用java代码静态扫描其他springboot项目中使用的redis地址
时间: 2023-08-31 21:20:22 浏览: 138
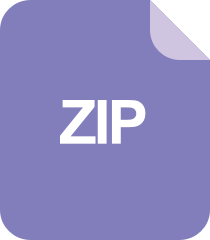
spring boot 集成redis代码
### 回答1:
可以使用Jedis工具类来扫描其他Spring Boot项目中使用的Redis地址。首先,需要在pom.xml文件中添加Jedis依赖,然后在Java代码中使用Jedis的API来连接Redis服务器并扫描Redis地址。具体实现可以参考以下代码:
```java
import redis.clients.jedis.Jedis;
import redis.clients.jedis.ScanParams;
import redis.clients.jedis.ScanResult;
public class RedisScanner {
public static void main(String[] args) {
// 连接Redis服务器
Jedis jedis = new Jedis("localhost", 6379);
// 扫描Redis地址
ScanParams params = new ScanParams().match("*");
String cursor = "";
do {
ScanResult<String> result = jedis.scan(cursor, params);
for (String key : result.getResult()) {
System.out.println(key);
}
cursor = result.getStringCursor();
} while (!cursor.equals(""));
// 关闭连接
jedis.close();
}
}
```
### 回答2:
要使用Java代码静态扫描其他Spring Boot项目中使用的Redis地址,可以采取以下步骤:
1. 引入必要的依赖:
在项目的pom.xml文件中添加Spring Boot和Redis相关的依赖,例如:
```xml
<!-- Spring Boot -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<!-- Redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 创建一个Java类来执行扫描操作:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.data.redis.RedisProperties;
import org.springframework.context.ApplicationContext;
@SpringBootApplication
public class RedisScanner {
public static void main(String[] args) {
ApplicationContext context = SpringApplication.run(RedisScanner.class, args);
RedisProperties redisProperties = context.getBean(RedisProperties.class);
System.out.println("Redis地址:" + redisProperties.getHost() + ":" + redisProperties.getPort());
}
}
```
3. 运行扫描程序:
在命令行或IDE中执行扫描程序的main方法,它将启动一个Spring Boot应用程序并输出Redis地址。
注意:确保已经正确配置了被扫描项目的Redis连接信息,扫描程序需要能够获取到该信息才能输出正确的Redis地址。
通过以上步骤,你可以使用Java代码静态扫描其他Spring Boot项目中使用的Redis地址,并将其输出到控制台或其他你需要的地方。
### 回答3:
要使用Java代码静态扫描其他Spring Boot项目中使用的Redis地址,可以使用以下步骤:
1. 导入相关的Java类库和依赖:首先需要导入Spring Boot的相关类库,如spring-boot-starter-data-redis,以及Redis的Java客户端类库,如jedis或Lettuce。
2. 获取其他项目的源代码:将其他Spring Boot项目的源代码进行获取,可以通过Git克隆或下载压缩包的方式获取。
3. 遍历源代码文件:通过递归方式遍历其他项目的源代码文件夹,可以使用Java的File类和FileUtils类。
4. 搜索Redis连接配置:在遍历的过程中,对每个文件进行解析,搜索Redis连接配置的相关代码。可以通过正则表达式匹配关键字,如"RedisTemplate"、"Redisson"等。
5. 提取Redis地址:根据找到的连接配置代码,提取其中的Redis地址信息。可以通过字符串截取、正则表达式或代码解析等方式提取Redis连接地址。
6. 封装结果:将提取到的Redis地址保存到一个列表或其他数据结构中,用于后续的使用或输出。
7. 输出结果:根据需求来定义如何输出结果,可以将结果保存到日志文件、数据库或控制台显示出来。
需要注意的是,以上步骤仅是一个大致的思路,具体的实现方式可能因项目的结构和要求而有所不同。在实现过程中,还需要考虑文件过滤、异常处理、性能调优等因素。
阅读全文
相关推荐




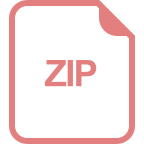
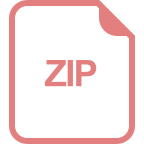
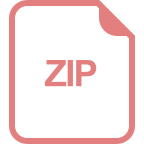
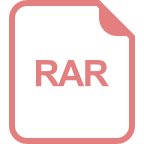
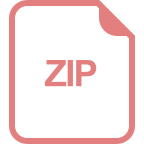
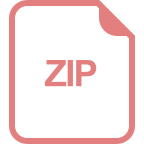
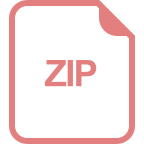
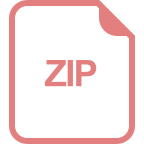
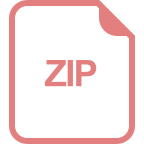
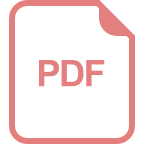
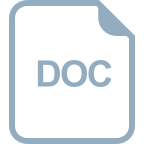
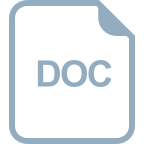