编写一个函数,统计字符串中字母、数字、空格和其他字符的个数,在主函数中调用该函数完成统计、字符串的输入,以及结果输出。c语言
时间: 2023-11-17 15:17:01 浏览: 101
以下是用C语言编写的统计字符串中字母、数字、空格和其他字符个数的代码:
```c
#include <stdio.h>
#include <ctype.h>
// 统计字符串中字母、数字、空格和其他字符的个数
void countChar(char *str, int *alphaCount, int *digitCount, int *spaceCount, int *otherCount) {
while(*str) {
if(isalpha(*str)) { // 判断是否为字母
(*alphaCount)++;
} else if(isdigit(*str)) { // 判断是否为数字
(*digitCount)++;
} else if(*str == ' ') { // 判断是否为空格
(*spaceCount)++;
} else { // 其他字符
(*otherCount)++;
}
str++;
}
}
int main() {
char str[100];
int alphaCount = 0, digitCount = 0, spaceCount = 0, otherCount = 0;
printf("请输入一个字符串:\n");
gets(str); // 读取字符串
countChar(str, &alphaCount, &digitCount, &spaceCount, &otherCount);
printf("字母个数:%d\n", alphaCount);
printf("数字个数:%d\n", digitCount);
printf("空格个数:%d\n", spaceCount);
printf("其他字符个数:%d\n", otherCount);
return 0;
}
```
在上面的代码中,使用了`isalpha()`、`isdigit()`和`isspace()`函数来判断字符是否为字母、数字和空格。`gets()`函数用于从标准输入流中读取字符串,但是不太安全,因为它无法防止缓冲区溢出,所以更好的方法是使用`fgets()`函数。
阅读全文
相关推荐
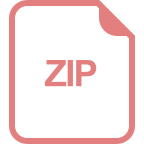















