用c语言编写一个函数,由实参传来一个字符串,统计此字符串中字母、空格、数字和其他字符的个数;在主函数中输入字符串,然后调用该函数进行统计并(在主函数中)输出结果
时间: 2023-11-17 15:11:50 浏览: 90
下面是实现该功能的 C 代码:
```c
#include <stdio.h>
#include <ctype.h>
void count_chars(char *str, int *alpha, int *space, int *digit, int *other)
{
int i = 0;
char ch;
while ((ch = str[i]) != '\0') {
if (isalpha(ch)) {
(*alpha)++;
} else if (isspace(ch)) {
(*space)++;
} else if (isdigit(ch)) {
(*digit)++;
} else {
(*other)++;
}
i++;
}
}
int main(void)
{
char str[100];
int alpha = 0, space = 0, digit = 0, other = 0;
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
count_chars(str, &alpha, &space, &digit, &other);
printf("Number of alphabetic characters: %d\n", alpha);
printf("Number of spaces: %d\n", space);
printf("Number of digits: %d\n", digit);
printf("Number of other characters: %d\n", other);
return 0;
}
```
在 `count_chars` 函数中,我们遍历字符串中的每个字符,使用 `isalpha`、`isspace` 和 `isdigit` 函数判断该字符属于哪一类,并将相应的计数器加一。
在 `main` 函数中,我们首先通过 `fgets` 函数从标准输入中读取一个字符串,然后调用 `count_chars` 函数统计该字符串中的各种字符的个数,并输出结果。
阅读全文
相关推荐
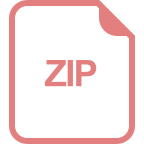















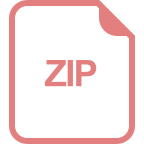