springboot3.3 集成 cxf
时间: 2024-09-11 22:18:59 浏览: 154
Spring Boot 3.3 集成 Apache CXF 是一种流行的集成方式,用于构建轻量级的、RESTful 和 SOAP Web 服务。Apache CXF 是一个开源的服务框架,它简化了 Web 服务的创建和消费。Spring Boot 通过其自动配置和 starter 依赖项大大简化了 CXF 的集成和配置。
集成步骤大致如下:
1. 添加依赖项:在项目的 pom.xml 或 build.gradle 文件中添加 Spring Boot 和 CXF 的依赖项。Spring Boot 会自动配置 CXF,提供一个默认的 `CxfEndpoint` 实例。
Maven pom.xml 示例:
```xml
<dependencies>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-spring-boot-starter-jaxws</artifactId>
<version>3.3.x</version> <!-- 替换为实际的版本号 -->
</dependency>
<!-- 其他依赖项 -->
</dependencies>
```
2. 创建 Web 服务接口和实现类:定义你的服务接口和实现类。CXF 将会使用这些类来创建 Web 服务。
示例:
```java
@WebService
public interface MyService {
String sayHello(String name);
}
@WebService(endpointInterface = "com.example.MyService")
public class MyServiceImpl implements MyService {
@Override
public String sayHello(String name) {
return "Hello, " + name;
}
}
```
3. 配置服务端点:使用 `@Endpoint` 注解来发布你的服务。
示例:
```java
@Service
@org.apache.cxf.jaxws.EndpointImpl
public class MyServicePublisher {
@Autowired
public MyServicePublisher(@Qualifier("myService") MyService myService) {
Endpoint.publish("/myService", myService);
}
}
```
4. 启动类:确保你的 Spring Boot 应用有一个主启动类,它使用 `@SpringBootApplication` 注解。
5. 启动和测试服务:启动 Spring Boot 应用,CXF 会自动配置并启动内嵌的服务器(如 Jetty 或 Tomcat),然后你可以通过端点访问你的 Web 服务。
测试示例:
```java
// 在测试类中
String address = "http://localhost:8080/myService";
MyService service = new MyServiceLocator().getMyService(address);
System.out.println(service.sayHello("World"));
```
以上步骤是集成 Spring Boot 和 CXF 的基本流程,具体实现可能还需要考虑安全性、拦截器、异常处理等高级功能。
阅读全文
相关推荐
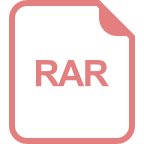
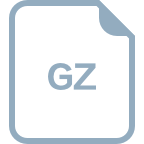
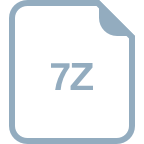
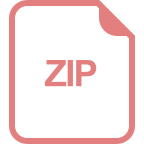



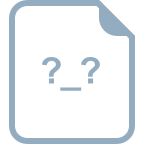
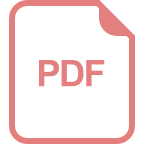
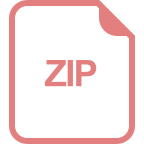
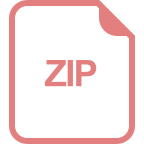
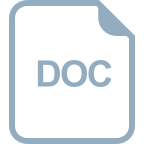
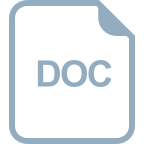
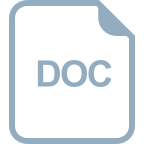



