C#搜索算法 哈希表查找
时间: 2024-10-25 19:01:25 浏览: 12
在C#中,哈希表(Hash Table 或 Dictionary)是一种高效的数据结构,它基于哈希函数来存储和检索数据。哈希表查找通常的时间复杂度是O(1),这意味着无论数据集大小,查找、插入和删除操作的速度都是常数级别的。
当你需要快速地根据键(key)获取值(value),哈希表是一个理想的选择。查找过程的基本步骤如下:
1. **计算哈希码**:通过键的哈希函数将键转换成一个整数哈希码,这个哈希码决定了值在哈希表中的位置。
2. **定位元素**:根据哈希码找到哈希表中对应的位置,这通常是数组的一个索引。
3. **检查冲突**:因为不同的键可能会有相同的哈希码(哈希冲突),所以需要在一个叫做哈希桶的范围内寻找真正的值。如果冲突,C#的Dictionary会采用链地址法或其他解决冲突的方式来进行查找。
例如,在C#中使用`Dictionary<TKey, TValue>`,你可以这样做:
```csharp
Dictionary<string, int> myHashTable = new Dictionary<string, int>();
// 插入元素
myHashTable.Add("apple", 4);
// 查找元素
int value;
if (myHashTable.TryGetValue("apple", out value))
{
Console.WriteLine($"Value of 'apple': {value}");
}
```
相关问题
c# dictionary查找算法
C#中的`Dictionary`是基于哈希表实现的数据结构,因此查找操作的时间复杂度为O(1)。下面是一个示例代码:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
Dictionary<string, int> dict = new Dictionary<string, int>();
dict.Add("apple", 1);
dict.Add("banana", 2);
dict.Add("orange", 3);
if (dict.ContainsKey("apple"))
{
int value = dict["apple"];
Console.WriteLine($"The value of 'apple' is {value}");
}
else
{
Console.WriteLine("The key 'apple' does not exist in the dictionary");
}
if (dict.ContainsKey("pear"))
{
int value = dict["pear"];
Console.WriteLine($"The value of 'pear' is {value}");
}
else
{
Console.WriteLine("The key 'pear' does not exist in the dictionary");
}
}
}
```
输出结果为:
```
The value of 'apple' is 1
The key 'pear' does not exist in the dictionary
```
可以看到,通过`Dictionary`的`ContainsKey`方法和索引访问,可以快速判断一个键值是否存在,并获取对应的值。
c# Dictionary查找算法
C#中的`Dictionary`是基于哈希表实现的数据结构,因此查找操作的时间复杂度为O(1)。下面是一个示例代码:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
Dictionary<string, int> dict = new Dictionary<string, int>();
dict.Add("apple", 1);
dict.Add("banana", 2);
dict.Add("orange", 3);
if (dict.ContainsKey("apple"))
{
int value = dict["apple"];
Console.WriteLine($"The value of 'apple' is {value}");
}
else
{
Console.WriteLine("The key 'apple' does not exist in the dictionary");
}
if (dict.ContainsKey("pear"))
{
int value = dict["pear"];
Console.WriteLine($"The value of 'pear' is {value}");
}
else
{
Console.WriteLine("The key 'pear' does not exist in the dictionary");
}
}
}
```
输出结果为:
```
The value of 'apple' is 1
The key 'pear' does not exist in the dictionary
```
可以看到,通过`Dictionary`的`ContainsKey`方法和索引访问,可以快速判断一个键值是否存在,并获取对应的值。
阅读全文
相关推荐
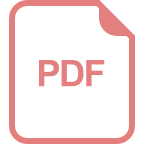
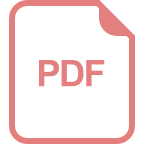
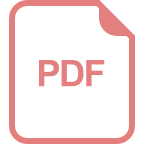
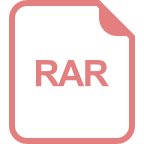
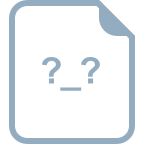
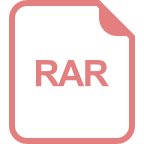
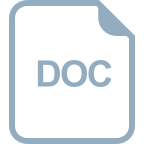
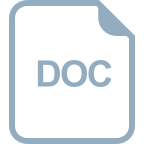
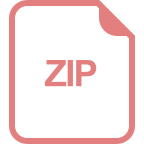
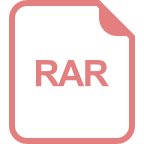
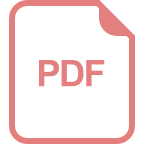
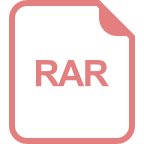
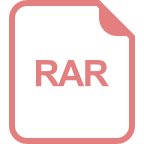
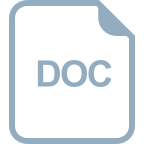
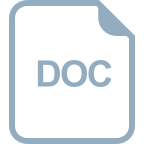
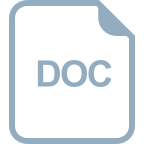