vue 圆上平均分布多个圆点,通过点击圆点让圆圈转起来
时间: 2024-09-20 22:13:30 浏览: 35
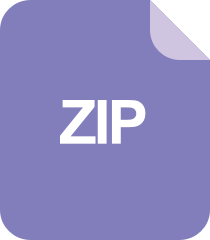
Canvas 鼠标点击生成小圆点
在Vue.js中,要实现这样的效果,你可以结合HTML、CSS和JavaScript(通常使用Vue的组件化思想)。首先,创建一个圆形元素,然后将多个圆点分布在主圆上。当用户点击某个圆点时,可以控制其关联的圆圈旋转。
1. HTML结构:
```html
<template>
<div class="circle-container">
<div v-for="(dot, index) in dots" :key="index" @click="rotateCircle(index)">
<div class="dot" :style="{ left: dot.x + 'px', top: dot.y + 'px' }"></div>
</div>
<div class="circle" ref="circle" @mouseenter="startRotation" @mouseleave="stopRotation">
<!-- 使用SVG或CSS transform来绘制动态旋转的圆 -->
</div>
</div>
</template>
```
2. CSS (假设SVG圆使用transform):
```css
.circle-container {
position: relative;
}
.dot {
position: absolute;
width: 10px;
height: 10px;
border-radius: 50%;
}
.circle {
position: absolute;
width: 100px;
height: 100px;
border-radius: 50%;
background-color: lightblue;
transition: transform 1s;
}
```
3. Vue.js 数据与方法:
```javascript
<script>
export default {
data() {
return {
dots: [
// 初始化一些随机分布在圆周上的坐标
{ x: Math.random() * 200, y: Math.sqrt(200 ** 2 - (Math.random() * 200) ** 2) },
... // 添加更多圆点
],
isRotating: false,
};
},
methods: {
rotateCircle(index) {
this.isRotating = true;
this.$nextTick(() => {
this.rotate(this.dots[index]);
});
},
rotate(dot) {
if (!this.isRotating) return;
const angle = (Math.atan2(dot.y, dot.x) + Math.PI / 2) % (2 * Math.PI);
this.$refs.circle.style.transform = `rotate(${angle * 180 / Math.PI}deg)`;
},
startRotation() {
this.isRotating = true;
},
stopRotation() {
this.isRotating = false;
// 清除动画
this.$refs.circle.style.transform = '';
},
},
};
</script>
```
在这个例子中,当你点击一个圆点时,对应的圆圈开始旋转,直到鼠标移开为止。注意这只是一个基础示例,实际项目中可能需要处理更复杂的交互逻辑和性能优化。
阅读全文
相关推荐
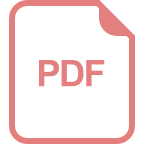
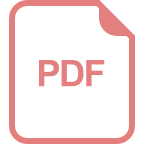
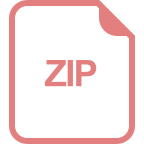
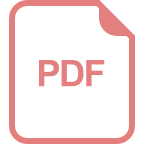
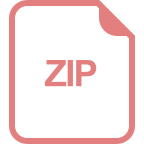
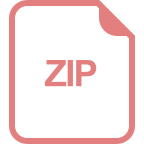
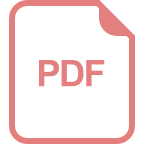
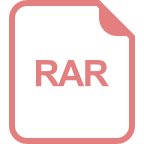
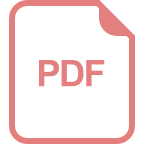
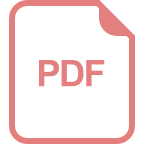
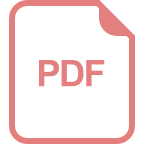
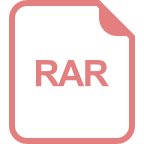