利用C语言链式队列: (1)初始化队列。 (2)入队。 (3)出队。 (4)取出队头元素
时间: 2024-10-21 21:08:45 浏览: 31
在C语言中,我们可以利用结构体和指针来实现链式队列。下面是对链式队列操作的简单介绍:
1. **初始化队列**(Enqueue Queue):
- 首先,我们需要定义一个队列节点结构体(Node),包含数据域(data)和指向下一个节点的指针(next)。
```c
struct Node {
int data;
struct Node* next;
};
```
初始化队列意味着创建一个新的空队列,可以设置一个队头指针指向NULL表示队列为空。
```c
struct Node* queue_head = NULL;
```
2. **入队**(enqueue):
- 当需要添加元素到队尾时,新建一个节点,将新节点的数据赋值,然后将其next指向前一个队尾节点,最后更新队尾指针。
```c
void enqueue(int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = queue_head;
queue_head = newNode;
} else {
printf("Memory allocation failed!\n");
}
}
```
3. **出队**(dequeue):
- 如果队列非空,出队操作会移除并返回队头元素,然后调整队头指针。如果队列为空,则无法出队。
```c
int dequeue() {
if (queue_head == NULL) {
return -1; // 或者抛出错误,表示队列已空
}
int removedValue = queue_head->data;
struct Node* temp = queue_head;
queue_head = queue_head->next;
free(temp); // 释放内存
return removedValue;
}
```
4. **取出队头元素**(Peek at front):
- 这与出队类似,但不会删除队首元素,只是查看它。
```c
int peek_front() {
if (queue_head == NULL) {
return -1; // 或者抛出异常
}
return queue_head->data;
}
```
阅读全文
相关推荐
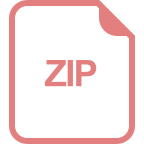
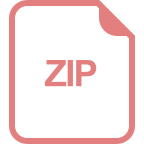
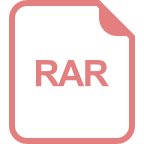

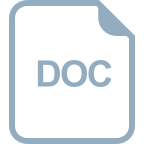
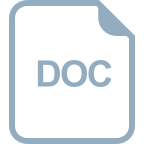

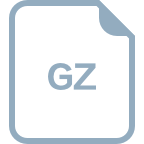
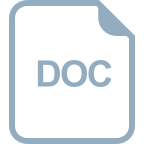









